python爬取一个人的微博
时间: 2023-07-04 16:05:44 浏览: 52
要爬取一个人的微博,需要使用微博的开放API,并且需要申请开发者账号和应用程序来获取API的访问权限。下面是通过Python代码来爬取一个人微博的基本步骤:
1. 安装 requests 和 json 库:
```
pip install requests
```
2. 获取微博开放API的访问令牌
首先需要注册成为微博开发者账号,然后创建应用程序,获取应用程序的 `client_id` 和 `client_secret`,用于后续获取访问令牌。
```python
import requests
def get_access_token(client_id, client_secret):
url = 'https://api.weibo.com/oauth2/access_token'
params = {
'grant_type': 'client_credentials',
'client_id': client_id,
'client_secret': client_secret,
}
response = requests.post(url, params=params)
return response.json()['access_token']
```
3. 获取用户的微博列表
使用获取微博列表的API:`https://api.weibo.com/2/statuses/user_timeline.json`,其中需要传入 `access_token` 和 `uid` 参数,`uid` 是需要爬取微博的用户的ID。
```python
def get_user_statuses(access_token, uid, count=100):
url = 'https://api.weibo.com/2/statuses/user_timeline.json'
params = {
'access_token': access_token,
'uid': uid,
'count': count,
}
response = requests.get(url, params=params)
return response.json()['statuses']
```
4. 解析微博内容
微博的内容包含在微博返回的 JSON 数据中,可以根据需要选择解析其中的字段。例如,可以解析微博的文本内容,发布时间,点赞数等信息。下面是一个简单的例子:
```python
def parse_statuses(statuses):
for status in statuses:
text = status['text'] # 微博文本内容
created_at = status['created_at'] # 发布时间
attitudes_count = status['attitudes_count'] # 点赞数
print(text, created_at, attitudes_count)
```
完整代码示例:
```python
import requests
def get_access_token(client_id, client_secret):
url = 'https://api.weibo.com/oauth2/access_token'
params = {
'grant_type': 'client_credentials',
'client_id': client_id,
'client_secret': client_secret,
}
response = requests.post(url, params=params)
return response.json()['access_token']
def get_user_statuses(access_token, uid, count=100):
url = 'https://api.weibo.com/2/statuses/user_timeline.json'
params = {
'access_token': access_token,
'uid': uid,
'count': count,
}
response = requests.get(url, params=params)
return response.json()['statuses']
def parse_statuses(statuses):
for status in statuses:
text = status['text'] # 微博文本内容
created_at = status['created_at'] # 发布时间
attitudes_count = status['attitudes_count'] # 点赞数
print(text, created_at, attitudes_count)
if __name__ == '__main__':
client_id = 'your_client_id'
client_secret = 'your_client_secret'
access_token = get_access_token(client_id, client_secret)
uid = 'your_uid'
statuses = get_user_statuses(access_token, uid)
parse_statuses(statuses)
```
需要注意的是,微博对于API的访问频率有限制,如果频繁访问可能会被封禁,因此建议适当控制访问频率。另外,微博的API文档中有详细的参数说明和返回字段说明,可以根据需要进行调整。
相关推荐
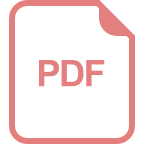
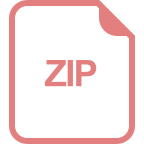
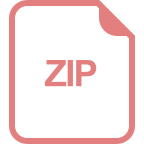









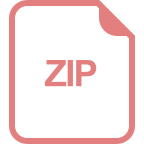
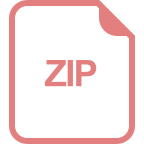
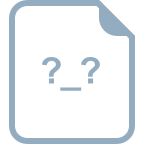
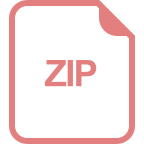
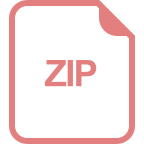
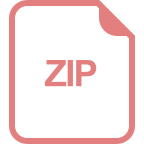