请使用C++字符串流结合常用STL容器完成以下功能: 统计这段文字的不重复的单词个数(即多次出现的单词只计为一个)输入一段不换行、含句号、逗号、双引号的英文文字。 输出 按单词首字母A-Z排序,一行一词输出小写字母和频数。
时间: 2023-06-16 15:06:42 浏览: 111
下面是C++的实现代码:
```c++
#include <iostream>
#include <sstream>
#include <string>
#include <map>
#include <set>
#include <algorithm>
using namespace std;
int main()
{
string text;
getline(cin, text);
// 去除标点符号
for (int i = 0; i < text.length(); i++) {
if (ispunct(text[i])) {
text.erase(i--, 1);
}
}
// 转换为小写
transform(text.begin(), text.end(), text.begin(), ::tolower);
// 统计单词频数
map<string, int> word_freq;
istringstream iss(text);
string word;
while (iss >> word) {
word_freq[word]++;
}
// 按单词首字母排序
set<string> words;
for (const auto& p : word_freq) {
words.insert(p.first);
}
// 输出结果
for (char c = 'a'; c <= 'z'; c++) {
for (const auto& word : words) {
if (word[0] == c) {
cout << word << " " << word_freq[word] << endl;
}
}
}
return 0;
}
```
解释:
首先读入一行文本,然后去除标点符号,并转换为小写字母。接着使用istringstream将文本分割为单词,统计每个单词的出现频数并保存在map容器word_freq中。然后使用set容器words保存所有不重复的单词,并按照首字母排序。最后按照字母顺序和单词频数输出结果。
阅读全文
相关推荐
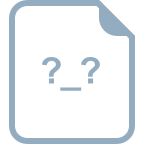
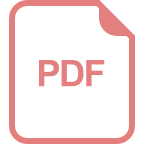
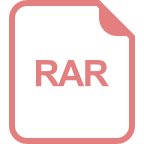















