python 连接mysql
时间: 2023-09-04 20:08:11 浏览: 39
在Python中连接MySQL需要使用MySQL官方提供的Python驱动程序(Python MySQL Connector)。首先,需要安装驱动程序。可以使用以下命令来安装MySQL Connector:
```
pip install mysql-connector-python
```
安装完成后,可以按照以下步骤连接MySQL:
1. 导入MySQL Connector模块:
```python
import mysql.connector
```
2. 使用`connect()`函数连接数据库:
```python
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="mydatabase"
)
```
在此示例中,我们连接名为“mydatabase”的MySQL数据库。请确保将“yourusername”和“yourpassword”替换为您的MySQL用户名和密码。
3. 创建游标对象:
```python
mycursor = mydb.cursor()
```
4. 执行SQL查询:
```python
mycursor.execute("SELECT * FROM customers")
```
在此示例中,我们执行名为“customers”的表上的SELECT查询。
5. 获取查询结果:
```python
myresult = mycursor.fetchall()
for x in myresult:
print(x)
```
在此示例中,我们使用`fetchall()`方法获取查询结果,并使用循环打印结果。
完整示例代码:
```python
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="mydatabase"
)
mycursor = mydb.cursor()
mycursor.execute("SELECT * FROM customers")
myresult = mycursor.fetchall()
for x in myresult:
print(x)
```
相关推荐
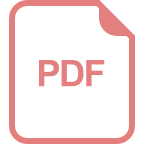



