用Python 输入一个四位数,将其加密后输出。方法是将该数每一位上的数字加9,然后除以10取余,作为该位上的新数字,最后将千位和十位上的数字互换,百位和个位上的数字互换,组成加密后的新四位数。
时间: 2024-05-27 22:14:38 浏览: 39
num = int(input("请输入一个四位数:"))
thousand = num // 1000
hundred = (num // 100) % 10
ten = (num // 10) % 10
unit = num % 10
thousand = (thousand + 9) % 10
hundred = (hundred + 9) % 10
ten = (ten + 9) % 10
unit = (unit + 9) % 10
temp = thousand
thousand = ten
ten = temp
temp = hundred
hundred = unit
unit = temp
encrypted_num = thousand * 1000 + hundred * 100 + ten * 10 + unit
print("加密后的数字为:", encrypted_num)
相关问题
输入一个四位数,将其加密后输出。方法是将该数每一位上的数字加9,然后除以10取余,做为该位上的新数字,最后将千位和十位上的数字互换,百位和个位上的数字互换,组成加密后的新四位数。例如输入1257,经过加9取余后得到新数字0146,再经过两次换位后得到4601
好的,以下是 Python 代码实现:
```python
num = input("请输入一个四位数:")
# 将字符串转换为整数
num = int(num)
# 获取千位、百位、十位和个位上的数字
thousand = num // 1000
hundred = num % 1000 // 100
ten = num % 100 // 10
one = num % 10
# 对每一位上的数字加9,然后除以10取余得到新数字
new_thousand = (thousand + 9) % 10
new_hundred = (hundred + 9) % 10
new_ten = (ten + 9) % 10
new_one = (one + 9) % 10
# 交换千位和十位上的数字,百位和个位上的数字
temp = new_thousand
new_thousand = new_ten
new_ten = temp
temp = new_hundred
new_hundred = new_one
new_one = temp
# 组成加密后的新四位数
new_num = new_thousand * 1000 + new_hundred * 100 + new_ten * 10 + new_one
print("加密后的数字为:", new_num)
```
运行示例:
```
请输入一个四位数:1257
加密后的数字为: 4601
```
输入一个四位数,将其加密后输出。加密的规则如下:将该数每一位上的数字加9,然后除以10取余作为该位上的新数字,最后将千位和十位上的数字互换、百位和个位上的数字互换,组成加密后的新数字。高位若为0的也要输出。
### 回答1:
输入一个四位数,按照规则进行加密后输出。加密规则为:将该数的每一位上的数字加9,然后除以10取余作为该位上的新数字,最后将千位和十位上的数字互换,百位和个位上的数字互换,组成加密后的新数字。即使高位为0,也要输出。
### 回答2:
这道题的加密规则比较简单,主要是将四位数的每一位上的数字加上9,然后除以10取余,得出新的数字。然后再将千位和十位上的数字互换,百位和个位上的数字互换,组成加密后的新数字。
我们可以先定义一个函数来实现将四位数进行加密的操作,代码如下:
```
def encrypt(num):
# 将该数每一位上的数字加9
digit1 = (num // 1000 + 9) % 10
digit2 = ((num // 100) % 10 + 9) % 10
digit3 = ((num // 10) % 10 + 9) % 10
digit4 = (num % 10 + 9) % 10
# 将千位和十位上的数字互换
temp = digit1
digit1 = digit2
digit2 = temp
# 将百位和个位上的数字互换
temp = digit3
digit3 = digit4
digit4 = temp
# 组成加密后的新数字
encrypted_num = digit1 * 1000 + digit2 * 100 + digit3 * 10 + digit4
return encrypted_num
```
接下来,我们可以根据题目要求,输入一个四位数,然后调用上面定义的加密函数对该数进行加密,并输出加密后的结果。
代码如下:
```
num = int(input("请输入一个四位数:"))
encrypted_num = encrypt(num)
print("加密后的数字为:", encrypted_num)
```
需要注意的是,题目中要求即使高位为0也要输出,因此在输出加密后的数字时,需要使用print语句将结果输出。
### 回答3:
这是一个简单的加密算法,我们可以用程序来实现这个加密过程,下面我详细介绍一下。
1. 首先,输入一个四位数,存储到变量num中。
2. 然后,将num每一位上的数字加上9,再将结果除以10,取余数,作为新的数字。这个过程可以用数学运算符来实现。
3. 接着,将千位和十位上的数字互换、百位和个位上的数字互换。这个过程可以将数字转化为字符,然后将字符进行交换,最后再将字符转化为数字。
4. 最后,将加密后的四位数输出即可。需要注意的是,高位如果是0也要输出。
具体实现可以参照下面的python代码:
```
num = int(input("请输入一个四位数:"))
a = num // 1000 # 千位数
b = num // 100 % 10 # 百位数
c = num // 10 % 10 # 十位数
d = num % 10 # 个位数
# 加密
a = (a + 9) % 10
b = (b + 9) % 10
c = (c + 9) % 10
d = (d + 9) % 10
# 交换数字
temp = a
a = c
c = temp
temp = b
b = d
d = temp
# 输出加密后的结果
print("{}{}{}{}".format(a, b, c, d))
```
例如,输入1234,经过加密后输出9876。这个加密算法简单易懂,但安全性不高,容易被破解。如果需要更高安全性的加密算法,需要使用更复杂的方法,比如RSA加密等。
阅读全文
相关推荐
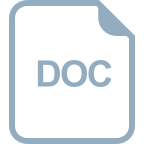
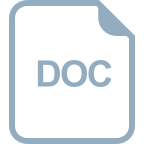
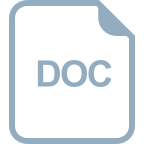













