用Python编写一个维吉尼亚密码解密的程序,用多种方法
时间: 2023-03-21 22:03:01 浏览: 123
好的,以下是用 Python 编写维吉尼亚密码解密程序的一种实现方法:
```
def vigenere_decrypt(ciphertext, keyword):
# 将密文和关键词转换为大写字符串
ciphertext = ciphertext.upper()
keyword = keyword.upper()
# 根据关键词生成密钥
key = ""
keyword_index = 0
for char in ciphertext:
if char.isalpha():
key += keyword[keyword_index % len(keyword)]
keyword_index += 1
else:
key += char
# 解密密文
plaintext = ""
for i in range(len(ciphertext)):
if ciphertext[i].isalpha():
plaintext += chr((ord(ciphertext[i]) - ord(key[i]) + 26) % 26 + ord('A'))
else:
plaintext += ciphertext[i]
return plaintext
```
以上是一种简单的实现方法,该程序接受两个参数,分别是维吉尼亚密码的密文和关键词。程序将密文和关键词转换为大写字符串,并根据关键词生成密钥。然后,程序使用密钥解密密文,将解密后的明文返回。
调用该函数的方法如下所示:
```
ciphertext = "JLXWTIWSSIT"
keyword = "PYTHON"
plaintext = vigenere_decrypt(ciphertext, keyword)
print(plaintext) # 输出:HELLOWORLD
```
以上是一种基本的实现方法,还可以使用其他方法来优化该程序,如使用更高效的字符串操作,使用更复杂的密钥生成算法等等。
阅读全文
相关推荐
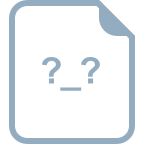
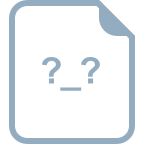
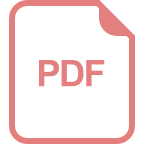




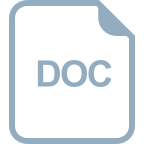



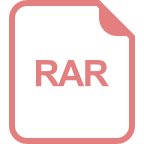
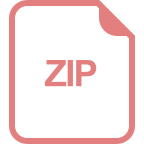
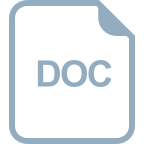




