基于Oracle.ManagedDataAccess.Client中的ArrayBindCount方法批量插入含有日期时间和string数据
时间: 2024-02-22 11:59:04 浏览: 27
可以使用OracleParameter对象和ArrayBindCount方法实现批量插入含有日期时间和string数据的操作。以下是示例代码:
```csharp
using Oracle.ManagedDataAccess.Client;
using System;
using System.Collections.Generic;
class Program
{
static void Main(string[] args)
{
string connectionString = "Data Source=your_data_source;User Id=your_user_id;Password=your_password;";
int batchSize = 1000; // 每批次插入的数据条数
DateTime[] dateTimeValues = new DateTime[] { DateTime.Now, DateTime.Now.AddDays(1), DateTime.Now.AddDays(2) };
string[] stringValues = new string[] { "value1", "value2", "value3" };
using (OracleConnection connection = new OracleConnection(connectionString))
{
connection.Open();
OracleCommand command = new OracleCommand();
command.Connection = connection;
command.CommandText = "INSERT INTO your_table (date_column, string_column) VALUES (:date_value, :string_value)";
command.CommandType = System.Data.CommandType.Text;
OracleParameter dateParameter = new OracleParameter("date_value", OracleDbType.Date);
OracleParameter stringParameter = new OracleParameter("string_value", OracleDbType.Varchar2);
command.Parameters.Add(dateParameter);
command.Parameters.Add(stringParameter);
int totalRows = dateTimeValues.Length;
int batchCount = (totalRows + batchSize - 1) / batchSize;
for (int i = 0; i < batchCount; i++)
{
int startRow = i * batchSize;
int endRow = Math.Min(startRow + batchSize, totalRows);
List<DateTime> dateTimeBatch = new List<DateTime>();
List<string> stringBatch = new List<string>();
for (int j = startRow; j < endRow; j++)
{
dateTimeBatch.Add(dateTimeValues[j]);
stringBatch.Add(stringValues[j]);
}
dateParameter.Value = dateTimeBatch.ToArray();
stringParameter.Value = stringBatch.ToArray();
command.ArrayBindCount = endRow - startRow;
int affectedRows = command.ExecuteNonQuery();
Console.WriteLine("{0} rows affected in batch {1}", affectedRows, i + 1);
}
}
}
}
```
在上面的代码中,我们将待插入的日期时间和string数据分批次进行插入,每批次插入的数据条数由batchSize变量指定。将日期时间和string数据分别存储在dateTimeValues和stringValues数组中,然后通过循环分批次进行插入,直到所有数据都插入完成。注意,每批次插入时需要设置ArrayBindCount属性,指定当前批次中待插入的数据条数。
相关推荐
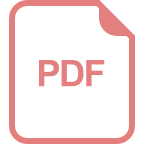














