Velocity 自定义指定模板路径static
时间: 2023-11-03 10:04:18 浏览: 36
如果您想要更加灵活地指定Velocity模板的路径,可以自定义一个模板加载器(`org.apache.velocity.runtime.resource.loader.ResourceLoader`),然后在Velocity的配置文件中使用该加载器。
以下是一个示例实现,展示了如何创建一个自定义的模板加载器:
```java
public class MyResourceLoader extends ResourceLoader {
private String basePath;
public void init(ExtendedProperties configuration) {
basePath = configuration.getString("base_path", "/path/to/templates");
}
public InputStream getResourceStream(String templateName) throws ResourceNotFoundException {
InputStream inputStream = null;
try {
File file = new File(basePath, templateName);
inputStream = new FileInputStream(file);
} catch (Exception e) {
throw new ResourceNotFoundException("Could not load template: " + templateName, e);
}
return inputStream;
}
public boolean isSourceModified(Resource resource) {
return false; // 不检查模板文件是否被修改
}
public long getLastModified(Resource resource) {
return 0; // 永远返回0,因为我们不检查模板文件的修改时间
}
}
```
在上面的示例中,我们创建了一个名为 `MyResourceLoader` 的自定义模板加载器。该加载器使用一个基准路径(`basePath`)来指定模板文件所在的目录。在 `getResourceStream` 方法中,我们将基准路径和模板文件名组合起来,然后使用 `FileInputStream` 来加载模板文件。在 `isSourceModified` 和 `getLastModified` 方法中,我们不进行任何检查,直接返回固定的值。
接下来,在Velocity的配置文件中,我们需要将自定义加载器配置为模板加载器。以下是一个示例配置文件:
```
resource.loader = myloader
myloader.resource.loader.class = com.example.MyResourceLoader
myloader.base_path = /path/to/templates
```
在上面的示例中,`resource.loader` 属性被设置为 `myloader`,表示我们使用自定义的模板加载器。`myloader.resource.loader.class` 属性被设置为 `com.example.MyResourceLoader`,表示我们使用 `MyResourceLoader` 类来加载模板。`myloader.base_path` 属性被设置为静态模板路径,例如 `/path/to/templates`。
通过以上配置,当使用Velocity引擎来渲染模板时,它会使用自定义的模板加载器来加载模板文件。例如,如果我们有一个名为 `mytemplate.vm` 的模板文件,可以使用以下代码来渲染它:
```
VelocityEngine engine = new VelocityEngine();
engine.setProperty("resource.loader", "myloader");
engine.setProperty("myloader.resource.loader.class", "com.example.MyResourceLoader");
engine.setProperty("myloader.base_path", "/path/to/templates");
engine.init();
Template template = engine.getTemplate("mytemplate.vm");
```
相关推荐
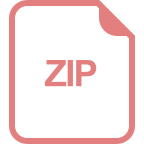
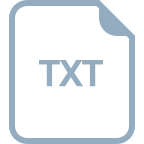














