Python使用线程池连接多个S7-1200实现读写
时间: 2023-07-11 10:46:17 浏览: 308
如果你需要同时连接多个S7-1200并实现读写,可以使用线程池来实现。线程池是一组预先创建的线程,可以用于执行多个并发任务。以下是一个示例代码,可以使用线程池连接多个S7-1200并从它们读取数据:
```python
import snap7
from concurrent.futures import ThreadPoolExecutor
# 定义连接S7-1200的函数
def connect_plc(ip, port):
plc = snap7.client.Client()
plc.connect(ip, port)
return plc
# 定义读取S7-1200数据的函数
def read_data(plc):
data = plc.read_area(snap7.types.Areas.DB, 1, 0, 10)
return data
# 定义S7-1200的IP地址和端口号
plc_ips = ['192.168.0.10', '192.168.0.20']
plc_ports = [102, 102]
# 创建线程池,并连接到S7-1200
with ThreadPoolExecutor(max_workers=len(plc_ips)) as executor:
plcs = list(executor.map(connect_plc, plc_ips, plc_ports))
# 读取S7-1200的数据
with ThreadPoolExecutor(max_workers=len(plcs)) as executor:
data_list = list(executor.map(read_data, plcs))
# 输出读取的数据
for i, data in enumerate(data_list):
print('Data from PLC', i+1, ':', data)
# 关闭连接
for plc in plcs:
plc.disconnect()
```
在这个示例中,我们首先定义了两个S7-1200的IP地址和端口号,并将它们存储在plc_ips和plc_ports变量中。然后,我们使用线程池连接到这些S7-1200,并将连接的结果存储在plcs列表中。接下来,我们使用线程池从每个S7-1200的DB1区域读取了10个字节的数据,并将其存储在data_list列表中。最后,我们输出读取的数据并关闭连接。
值得注意的是,我们使用max_workers参数来设置线程池的最大工作线程数。在这个示例中,我们设置它为连接的S7-1200数量。这样可以确保我们的程序不会创建过多的线程,从而导致系统资源不足。
阅读全文
相关推荐
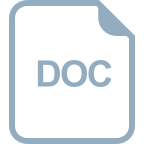
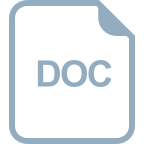
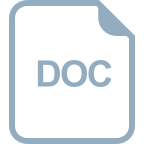

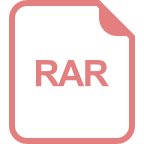
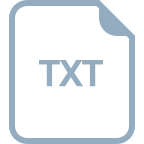
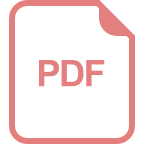
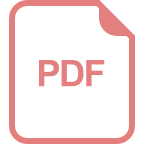
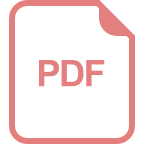
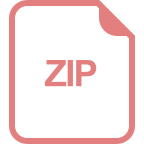
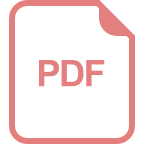
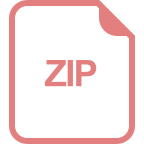
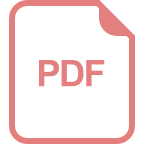
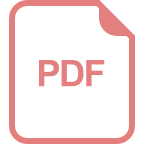
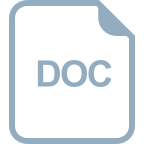
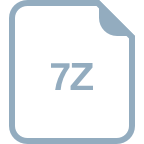
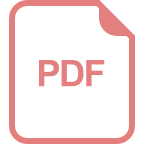
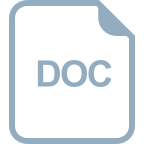