如何用c加加设计一个小型商品管理系统,设计要求 基本功能:商品信息管理 b) 增加商品价格信息 c) 删除商品价格信息 d) 修改商品价格信息 e) 查询商品价格 f) 商品价格排行浏览
时间: 2024-03-23 22:39:45 浏览: 17
首先,我们可以设计一个包含商品信息的结构体,如下所示:
```c++
struct Goods{
int id; // 商品编号
string name; // 商品名称
string description; // 商品描述
double price; // 商品价格
};
```
然后,我们可以设计一个商品管理类,其中包含增加、删除、修改、查询和排行浏览等功能。具体实现如下:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
// 商品信息结构体
struct Goods{
int id; // 商品编号
string name; // 商品名称
string description; // 商品描述
double price; // 商品价格
};
class GoodsManager{
public:
// 增加商品信息
void addGoods(const Goods& goods){
goodsList.push_back(goods);
}
// 删除商品信息
void deleteGoods(int id){
for (auto it = goodsList.begin(); it != goodsList.end(); ++it){
if (it->id == id){
goodsList.erase(it);
break;
}
}
}
// 修改商品价格信息
void modifyGoodsPrice(int id, double price){
for (auto it = goodsList.begin(); it != goodsList.end(); ++it){
if (it->id == id){
it->price = price;
break;
}
}
}
// 查询商品价格
double queryGoodsPrice(int id){
for (auto it = goodsList.begin(); it != goodsList.end(); ++it){
if (it->id == id){
return it->price;
}
}
return -1;
}
// 商品价格排行浏览
void browseGoodsByPrice(){
sort(goodsList.begin(), goodsList.end(),
[](const Goods& a, const Goods& b) -> bool{
return a.price > b.price;
});
for (const auto& goods : goodsList){
cout << goods.name << " : " << goods.price << endl;
}
}
private:
vector<Goods> goodsList; // 商品列表
};
```
使用示例:
```c++
int main(){
GoodsManager gm;
// 增加商品信息
Goods g1 = {1, "商品1", "这是商品1的描述", 10.0};
gm.addGoods(g1);
// 删除商品信息
gm.deleteGoods(1);
// 修改商品价格信息
gm.modifyGoodsPrice(1, 20.0);
// 查询商品价格
double price = gm.queryGoodsPrice(1);
cout << "商品1的价格为:" << price << endl;
// 商品价格排行浏览
gm.browseGoodsByPrice();
return 0;
}
```
相关推荐
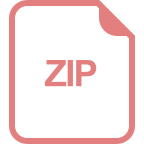
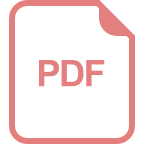














