根据类图,实现java代码:@startuml class BankAccount { -accountNumber : String -balance : double -ownerName : String +BankAccount(accountNumber:String, ownerName:String) +getAccountNumber():String +getBalance():double +getOwnerName():String +deposit(amount:double):void +withdraw(amount:double):void } class BankAccountDAO { -accounts : Map<String, BankAccount> -fileName : String +BankAccountDAO(fileName:String) +getAccount(accountNumber:String):BankAccount +getAllAccounts():List<BankAccount> +addAccount(account:BankAccount):void +updateAccount(account:BankAccount):void +deleteAccount(accountNumber:String):void -saveToFile():void } BankAccount -> BankAccountDAO @enduml
时间: 2024-02-01 10:13:48 浏览: 26
```java
import java.util.*;
class BankAccount {
private String accountNumber;
private double balance;
private String ownerName;
public BankAccount(String accountNumber, String ownerName) {
this.accountNumber = accountNumber;
this.ownerName = ownerName;
this.balance = 0.0;
}
public String getAccountNumber() {
return this.accountNumber;
}
public double getBalance() {
return this.balance;
}
public String getOwnerName() {
return this.ownerName;
}
public void deposit(double amount) {
this.balance += amount;
}
public void withdraw(double amount) {
if (balance >= amount) {
this.balance -= amount;
} else {
System.out.println("Insufficient funds!");
}
}
}
class BankAccountDAO {
private Map<String, BankAccount> accounts;
private String fileName;
public BankAccountDAO(String fileName) {
this.accounts = new HashMap<>();
this.fileName = fileName;
// TODO: load accounts from file into accounts map
}
public BankAccount getAccount(String accountNumber) {
return accounts.get(accountNumber);
}
public List<BankAccount> getAllAccounts() {
return new ArrayList<>(accounts.values());
}
public void addAccount(BankAccount account) {
accounts.put(account.getAccountNumber(), account);
saveToFile();
}
public void updateAccount(BankAccount account) {
accounts.replace(account.getAccountNumber(), account);
saveToFile();
}
public void deleteAccount(String accountNumber) {
accounts.remove(accountNumber);
saveToFile();
}
private void saveToFile() {
// TODO: write accounts map to file
}
}
```
相关推荐
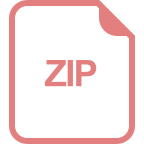
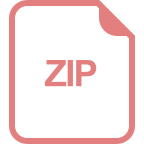















