编写程序从键盘输入10个整数,对前一半元素升序排序,对后一半元素降序排序,要求用c语言
时间: 2023-08-23 17:15:02 浏览: 99
好的,以下是使用 C 语言的代码实现:
```c
#include <stdio.h>
int main() {
// 从键盘输入10个整数
int num_list[10];
for (int i = 0; i < 10; i++) {
printf("请输入第%d个整数:", i+1);
scanf("%d", &num_list[i]);
}
// 对前一半元素升序排序
int first_half[5];
for (int i = 0; i < 5; i++) {
first_half[i] = num_list[i];
}
for (int i = 0; i < 5; i++) {
for (int j = i+1; j < 5; j++) {
if (first_half[i] > first_half[j]) {
int temp = first_half[i];
first_half[i] = first_half[j];
first_half[j] = temp;
}
}
}
// 对后一半元素降序排序
int second_half[5];
for (int i = 0; i < 5; i++) {
second_half[i] = num_list[i+5];
}
for (int i = 0; i < 5; i++) {
for (int j = i+1; j < 5; j++) {
if (second_half[i] < second_half[j]) {
int temp = second_half[i];
second_half[i] = second_half[j];
second_half[j] = temp;
}
}
}
// 合并前后两半
for (int i = 0; i < 5; i++) {
num_list[i] = first_half[i];
}
for (int i = 0; i < 5; i++) {
num_list[i+5] = second_half[i];
}
// 打印排序后的结果
printf("排序后的结果为:");
for (int i = 0; i < 10; i++) {
printf("%d ", num_list[i]);
}
printf("\n");
return 0;
}
```
运行以上代码后,在命令行中输入10个整数,程序会自动对前一半元素升序排序,后一半元素降序排序,并输出排序后的结果。
阅读全文
相关推荐



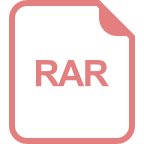













