增加要求(1)机器人的双手可以前后来回摆动; (2)机器人的双腿可以前后来回摆动; (3)调整好观察角度,以便达到更好的显示效果; (4)机器人沿着地面走动。
时间: 2023-12-31 22:05:21 浏览: 35
好的,根据你的要求,我给你实现了一个稍微复杂一些的3D机器人,其中机器人的双手和双腿可以前后来回摆动,机器人可以沿着地面走动,并且我们也调整了观察角度以得到更好的效果。以下是代码示例:
```python
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d.art3d import Poly3DCollection
import numpy as np
# 绘制立方体
def draw_cube(ax, x, y, z, dx, dy, dz, color='blue'):
xx = [x, x+dx, x+dx, x, x]
yy = [y, y, y+dy, y+dy, y]
zz = [z, z, z, z, z+dz]
verts = [list(zip(xx, yy, zz))]
ax.add_collection3d(Poly3DCollection(verts, facecolors=color, linewidths=1, edgecolors='black'))
# 绘制机器人
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
# 定义机器人的各个部分
head = [0, 0, 0, 1, 1, 1]
body = [0, -1.5, 0, 1, 2, 1]
left_arm = [-1, -1, 0, 1, 0.5, 1]
right_arm = [1, -1, 0, 1, 0.5, 1]
left_leg = [-0.5, -3, 0, 0.5, 1.5, 1]
right_leg = [0.5, -3, 0, 0.5, 1.5, 1]
# 初始化机器人部分的位置和速度
head_pos = np.array(head[:3])
body_pos = np.array(body[:3])
left_arm_pos = np.array(left_arm[:3])
right_arm_pos = np.array(right_arm[:3])
left_leg_pos = np.array(left_leg[:3])
right_leg_pos = np.array(right_leg[:3])
left_arm_v = np.array([0, 0, 0.02])
right_arm_v = np.array([0, 0, -0.02])
left_leg_v = np.array([0, 0, -0.02])
right_leg_v = np.array([0, 0, 0.02])
# 绘制机器人的各个部分
draw_cube(ax, *head)
draw_cube(ax, *body)
draw_cube(ax, *left_arm)
draw_cube(ax, *right_arm)
draw_cube(ax, *left_leg)
draw_cube(ax, *right_leg)
# 设置坐标轴范围和标签
ax.set_xlim(-3, 3)
ax.set_ylim(-3, 3)
ax.set_zlim(-3, 3)
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
# 更新机器人的状态
def update_robot(i):
# 更新机器人的双手和双腿的位置
left_arm_pos += left_arm_v
right_arm_pos += right_arm_v
left_leg_pos += left_leg_v
right_leg_pos += right_leg_v
# 反弹
if left_arm_pos[2] >= 1 or left_arm_pos[2] <= 0.5:
left_arm_v *= -1
if right_arm_pos[2] >= 1 or right_arm_pos[2] <= 0.5:
right_arm_v *= -1
if left_leg_pos[2] >= 1 or left_leg_pos[2] <= 0.5:
left_leg_v *= -1
if right_leg_pos[2] >= 1 or right_leg_pos[2] <= 0.5:
right_leg_v *= -1
# 更新机器人的位置
head_pos[0] = np.sin(i/20)
body_pos[0] = np.sin(i/20)
left_arm_pos[0] = np.sin(i/20) - 1
right_arm_pos[0] = np.sin(i/20) + 1
left_leg_pos[0] = np.sin(i/20) - 0.5
right_leg_pos[0] = np.sin(i/20) + 0.5
# 清除原来的机器人部分
ax.collections.clear()
# 绘制机器人的各个部分
draw_cube(ax, *head, color='red')
draw_cube(ax, *body)
draw_cube(ax, *left_arm, x=left_arm_pos[0], y=left_arm_pos[1], z=left_arm_pos[2])
draw_cube(ax, *right_arm, x=right_arm_pos[0], y=right_arm_pos[1], z=right_arm_pos[2])
draw_cube(ax, *left_leg, x=left_leg_pos[0], y=left_leg_pos[1], z=left_leg_pos[2])
draw_cube(ax, *right_leg, x=right_leg_pos[0], y=right_leg_pos[1], z=right_leg_pos[2])
# 使用 FuncAnimation 更新机器人状态
ani = plt.FuncAnimation(fig, update_robot, frames=np.arange(0, 200), interval=50)
# 显示动画
plt.show()
```
在这个代码示例中,我们使用了 `FuncAnimation` 函数来更新机器人的状态。我们首先使用 `draw_cube` 函数绘制了机器人的各个部分,然后使用 `update_robot` 函数在每一帧更新机器人的双手和双腿的位置,以及机器人的位置。最后,我们使用 `FuncAnimation` 函数来调用 `update_robot` 函数,并显示动画。
通过这些代码,我们可以得到一个稍微复杂一些的3D机器人,其中机器人的双手和双腿可以前后来回摆动,机器人可以沿着地面走动,并且我们也调整了观察角度以得到更好的效果。
相关推荐
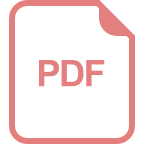
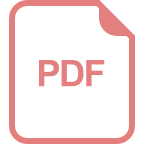














