用c语言从文件中读入任意一篇英文文件到链表
时间: 2023-08-20 22:04:05 浏览: 99
好的,我可以帮你解决这个问题。下面是一个简单的代码示例,它可以从文件中读取英文文本,并将每个单词存储到链表中。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define MAX_WORD_SIZE 50
typedef struct node {
char word[MAX_WORD_SIZE];
int count;
struct node *next;
} Node;
Node *createNode(char *word) {
Node *newNode = (Node *) malloc(sizeof(Node));
if (newNode == NULL) {
printf("Error: Unable to allocate memory for new node.\n");
exit(1);
}
strncpy(newNode->word, word, MAX_WORD_SIZE);
newNode->count = 1;
newNode->next = NULL;
return newNode;
}
void insertNode(Node **head, char *word) {
Node *current = *head;
Node *previous = NULL;
while (current != NULL && strcmp(word, current->word) > 0) {
previous = current;
current = current->next;
}
if (current != NULL && strcmp(word, current->word) == 0) {
current->count++;
return;
}
Node *newNode = createNode(word);
if (previous == NULL) {
newNode->next = *head;
*head = newNode;
} else {
newNode->next = current;
previous->next = newNode;
}
}
void printList(Node *head) {
while (head != NULL) {
printf("%s: %d\n", head->word, head->count);
head = head->next;
}
}
void destroyList(Node *head) {
Node *current = head;
while (current != NULL) {
Node *temp = current;
current = current->next;
free(temp);
}
}
int main(int argc, char *argv[]) {
if (argc < 2) {
printf("Error: Must specify input file.\n");
return 1;
}
char *filename = argv[1];
FILE *file = fopen(filename, "r");
if (file == NULL) {
printf("Error: Unable to open input file.\n");
return 1;
}
Node *head = NULL;
char word[MAX_WORD_SIZE];
int i = 0;
int c;
while ((c = fgetc(file)) != EOF) {
if (isalpha(c)) {
word[i++] = tolower(c);
} else if (i > 0) {
word[i] = '\0';
insertNode(&head, word);
i = 0;
}
}
if (i > 0) {
word[i] = '\0';
insertNode(&head, word);
}
fclose(file);
printList(head);
destroyList(head);
return 0;
}
```
这个代码示例使用 createNode 函数来创建新节点,insertNode 函数将新节点插入链表中,并按字母顺序排列。printList 函数打印链表中的每个单词及其出现次数,destroyList 函数释放链表内存。
阅读全文
相关推荐







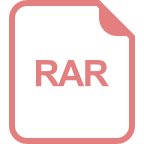
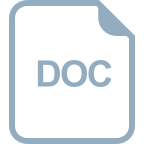
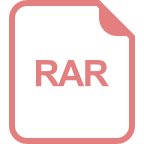


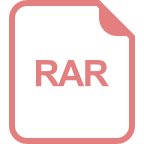
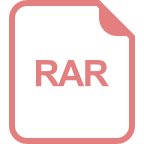
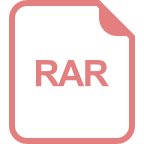
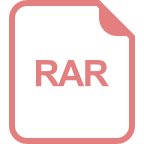