c++ 前置声明的类在一个命名空间中
时间: 2024-05-03 18:19:45 浏览: 9
是可以的。可以在一个命名空间中前置声明一个类,然后在另一个命名空间或者同一个命名空间的其他地方定义这个类。下面是一个示例代码:
```
namespace MyNamespace {
class MyClass; // 前置声明MyClass
}
namespace AnotherNamespace {
// 在另一个命名空间中定义MyClass
class MyNamespace::MyClass {
// 类成员定义
};
}
```
在上面的代码中,我们在`MyNamespace`中前置声明了`MyClass`类,然后在`AnotherNamespace`中定义了`MyNamespace::MyClass`类。注意,类的定义必须包含它所属的命名空间的限定符(`MyNamespace::`)。这样做可以避免不同命名空间中的类名称冲突。
相关问题
c++前置声明命名空间
在 C++ 中,可以使用前置声明来避免循环引用问题,同时可以使用命名空间来避免命名冲突。下面是示例代码:
```c++
// 前置声明命名空间
namespace my_namespace {
class MyClass; // 前置声明
}
// 使用命名空间中的类
void func(my_namespace::MyClass& obj) {
// ...
}
// 定义命名空间中的类
namespace my_namespace {
class MyClass {
// ...
};
}
```
在这个示例中,我们使用了命名空间 `my_namespace` 来定义一个类 `MyClass`,并使用前置声明来避免 `func()` 函数中的循环引用问题。
C++中 word这个类在哪个命名空间?
C++中并没有名为 word 的标准库类,word 可能是自定义的类。如果你是指 Microsoft Office Word 文件中的单词,那么需要使用 Microsoft Office Word 的 COM 接口来操作。如果你是指字符串中的一个单词,那么可以使用标准库中的 string 类来处理字符串,这个类定义在 std 命名空间中。
相关推荐
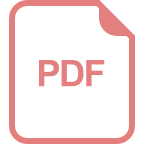












