C语言编写一个函数输入一个字符串,将字符 串中的*全部移动到字符串的尾部
时间: 2023-08-31 21:22:33 浏览: 176
### 回答1:
可以使用如下代码实现:int len = strlen(str);
int i, j; for(i=0, j=0; i<len; i++)
{
if(str[i] != '*')
{
str[j] = str[i];
j++;
}
} str[j] = '\0'; for(i=j; i<len; i++)
{
if(str[i] == '*')
{
str[j] = '*';
j++;
}
}
### 回答2:
可以编写一个函数,实现输入一个字符串,将字符串中的*全部移动到字符串的尾部。具体步骤如下:
1. 首先定义一个函数,接收一个字符串作为参数。
2. 创建一个新的字符串,用于存储移动后的结果。
3. 使用一个循环遍历原字符串中的每个字符。
4. 判断当前字符是否为*,如果是*则将其放到新的字符串的尾部。
5. 不是*的字符则直接放到新的字符串中。
6. 最后返回移动后的新字符串。
以下是示例代码:
```c
#include <stdio.h>
void moveStarsToEnd(char* str) {
// 创建一个新的字符串,用于存储移动后的结果
char new_str[100];
int count = 0;
// 遍历原字符串中的每个字符
for (int i = 0; str[i] != '\0'; i++) {
// 判断当前字符是否为*
if (str[i] == '*') {
// 将*放到新的字符串的尾部
new_str[count] = str[i];
count++;
}
}
// 将剩余的字符放到新的字符串中
for (int i = 0; str[i] != '\0'; i++) {
if (str[i] != '*') {
new_str[count] = str[i];
count++;
}
}
// 添加字符串结束符
new_str[count] = '\0';
// 打印移动后的字符串
printf("移动后的字符串:%s\n", new_str);
}
int main() {
char str[100];
// 输入字符串
printf("请输入字符串:");
scanf("%s", str);
// 调用移动函数
moveStarsToEnd(str);
return 0;
}
```
通过以上函数,我们可以实现输入一个字符串,并将其中的*全部移动到字符串的尾部。例如,输入字符串"ab*c*def*g",则移动后的字符串为"abcdefg**"。
### 回答3:
下面是一个C语言编写的函数,实现将字符串中的*全部移动到字符串的尾部:
```c
#include <stdio.h>
#include <string.h>
void moveCharToEnd(char *str) {
int len = strlen(str);
int count = 0;
// 统计字符串中的*的个数
for (int i = 0; i < len; i++) {
if (str[i] == '*') {
count++;
}
}
// 将字符串中的非*字符移到前面
int index = 0;
for (int i = 0; i < len; i++) {
if (str[i] != '*') {
str[index] = str[i];
index++;
}
}
// 在字符串末尾添加*的个数个*
for (int i = 0; i < count; i++) {
str[index] = '*';
index++;
}
// 字符串末尾添加结束符号
str[index] = '\0';
}
int main() {
char str[100];
printf("请输入字符串:");
scanf("%s", str);
moveCharToEnd(str);
printf("移动后的字符串: %s\n", str);
return 0;
}
```
这段代码首先计算字符串中的*的个数,然后将字符串中的非*字符移到字符串的前面,最后在字符串的末尾添加*的个数个*。接着在主函数中输入一个字符串,调用moveCharToEnd函数进行移动操作,并打印移动后的字符串。
阅读全文
相关推荐
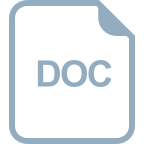
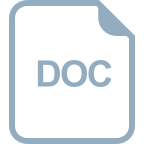
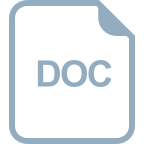















