html中 input框获取后台model中封装的对象的值
时间: 2023-08-11 18:05:28 浏览: 104
如果你想要使用input框获取后台model中封装的对象的值,需要先将后台的数据传递到前端页面中。具体的实现方式可能会因为你使用的后端框架和前端框架而有所不同,以下是一些常见的实现方式:
1. 通过后端框架的模板引擎将后台数据渲染到前端页面中,然后在input框中使用模板引擎的语法将数据绑定到input框中。例如,在使用Java的Spring框架时,可以使用Thymeleaf模板引擎来实现:
```html
<form action="/submit" method="post" th:object="${user}">
<input type="text" th:field="*{username}">
<input type="password" th:field="*{password}">
<button type="submit">提交</button>
</form>
```
以上代码中,我们使用Thymeleaf模板引擎将后台的user对象渲染到前端页面中,并通过`th:field`指令将user对象的属性与input框进行绑定。
2. 通过后端框架的接口将后台数据以json格式传递到前端页面中,然后在JavaScript中将json数据解析成对象,并将对象的属性绑定到input框中。例如,在使用Python的Django框架时,可以使用Django的内置视图函数`JsonResponse`将后台的数据以json格式返回到前端页面中:
```python
# views.py
from django.http import JsonResponse
def user(request):
user = {'username': 'admin', 'password': '123456'}
return JsonResponse(user)
```
```html
<!-- index.html -->
<form onsubmit="return false;">
<input type="text" id="username">
<input type="password" id="password">
<button onclick="submitForm()">提交</button>
</form>
<script>
fetch('/user')
.then(response => response.json())
.then(user => {
document.getElementById("username").value = user.username;
document.getElementById("password").value = user.password;
});
function submitForm() {
// 获取输入框中的值
var username = document.getElementById("username").value;
var password = document.getElementById("password").value;
// 在这里可以将用户名和密码发送到后端服务器
}
</script>
```
以上代码中,我们使用Django的`JsonResponse`函数将后台的user对象以json格式返回到前端页面中。在JavaScript中,我们使用`fetch`函数发送请求,并在回调函数中将json数据解析成user对象,并将user对象的属性绑定到input框中。在`submitForm`方法中,我们可以将input框中的值发送到后端服务器。
相关推荐
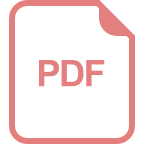
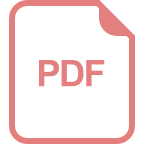














