设计异形迷宫,不同于传统的方形迷宫,异形迷宫 中每个迷宫单元是由相同的多边形组成,如图,是 一个六边形迷宫,每一个单元格与其它单元格最多 有六个通道可以连接。 1)自动生成并走出迷宫。 2)迷宫单元的边数由用户输入确定。c++
时间: 2024-05-10 18:18:47 浏览: 142
为了生成异形迷宫,我们可以使用递归分割法。首先,我们需要定义一个表示迷宫单元格的类,其中包含了单元格的坐标、边数以及与其相邻的单元格。
```
class Cell {
public:
int x;
int y;
int numSides;
vector<Cell*> neighbors;
bool visited;
Cell(int _x, int _y, int _numSides) {
x = _x;
y = _y;
numSides = _numSides;
visited = false;
}
};
```
接下来,我们需要定义一个生成迷宫的函数。该函数接受两个参数,分别是迷宫的行数和列数。在函数中,我们首先创建一个二维数组,用于存储迷宫单元格。然后,我们从左上角的单元格开始,使用递归分割法生成迷宫。
```
void generateMaze(int numRows, int numCols, int numSides) {
// Create maze cells
vector<vector<Cell*>> maze(numRows, vector<Cell*>(numCols));
for (int i = 0; i < numRows; i++) {
for (int j = 0; j < numCols; j++) {
maze[i][j] = new Cell(i, j, numSides);
}
}
// Generate maze
generateMazeRecursive(maze, 0, 0, numRows - 1, numCols - 1);
}
```
在递归分割法中,我们首先确定一个矩形区域,然后在该区域内随机选择一条竖直或水平的边,将矩形分成两部分。接着,我们递归地对两个子区域进行相同的操作,直到矩形区域的长度或宽度为1为止。
```
void generateMazeRecursive(vector<vector<Cell*>>& maze, int x1, int y1, int x2, int y2) {
if (x2 - x1 < 2 || y2 - y1 < 2) {
return;
}
// Determine orientation of wall to remove
bool isHorizontal = (x2 - x1 > y2 - y1);
int wallX = isHorizontal ? randomInt(x1, x2 - 1) : randomInt(x1, x2);
int wallY = isHorizontal ? randomInt(y1, y2) : randomInt(y1, y2 - 1);
// Remove wall
if (isHorizontal) {
maze[wallX][wallY]->numSides--;
maze[wallX + 1][wallY]->numSides--;
} else {
maze[wallX][wallY]->numSides--;
maze[wallX][wallY + 1]->numSides--;
}
// Recurse on sub-rectangles
if (isHorizontal) {
generateMazeRecursive(maze, x1, y1, x2, wallY);
generateMazeRecursive(maze, x1, wallY + 1, x2, y2);
} else {
generateMazeRecursive(maze, x1, y1, wallX, y2);
generateMazeRecursive(maze, wallX + 1, y1, x2, y2);
}
}
```
最后,我们需要实现一个函数来解决迷宫。该函数可以采用深度优先搜索或广度优先搜索算法。在遍历迷宫单元格时,我们需要考虑每个单元格的边数,以确保只遍历到未被访问过的相邻单元格。
```
void solveMaze(vector<vector<Cell*>>& maze) {
stack<Cell*> stack;
Cell* start = maze[0][0];
Cell* end = maze[maze.size() - 1][maze[0].size() - 1];
stack.push(start);
start->visited = true;
while (!stack.empty()) {
Cell* current = stack.top();
if (current == end) {
break;
}
bool foundUnvisitedNeighbor = false;
for (int i = 0; i < current->numSides; i++) {
Cell* neighbor = current->neighbors[i];
if (!neighbor->visited) {
stack.push(neighbor);
neighbor->visited = true;
foundUnvisitedNeighbor = true;
break;
}
}
if (!foundUnvisitedNeighbor) {
stack.pop();
}
}
// Print solution
while (!stack.empty()) {
Cell* current = stack.top();
cout << "(" << current->x << ", " << current->y << ")" << endl;
stack.pop();
}
}
```
完整代码如下:
```
#include <iostream>
#include <vector>
#include <stack>
#include <cstdlib>
#include <ctime>
using namespace std;
class Cell {
public:
int x;
int y;
int numSides;
vector<Cell*> neighbors;
bool visited;
Cell(int _x, int _y, int _numSides) {
x = _x;
y = _y;
numSides = _numSides;
visited = false;
}
};
int randomInt(int lower, int upper) {
return (rand() % (upper - lower + 1)) + lower;
}
void generateMazeRecursive(vector<vector<Cell*>>& maze, int x1, int y1, int x2, int y2) {
if (x2 - x1 < 2 || y2 - y1 < 2) {
return;
}
// Determine orientation of wall to remove
bool isHorizontal = (x2 - x1 > y2 - y1);
int wallX = isHorizontal ? randomInt(x1, x2 - 1) : randomInt(x1, x2);
int wallY = isHorizontal ? randomInt(y1, y2) : randomInt(y1, y2 - 1);
// Remove wall
if (isHorizontal) {
maze[wallX][wallY]->numSides--;
maze[wallX + 1][wallY]->numSides--;
} else {
maze[wallX][wallY]->numSides--;
maze[wallX][wallY + 1]->numSides--;
}
// Recurse on sub-rectangles
if (isHorizontal) {
generateMazeRecursive(maze, x1, y1, x2, wallY);
generateMazeRecursive(maze, x1, wallY + 1, x2, y2);
} else {
generateMazeRecursive(maze, x1, y1, wallX, y2);
generateMazeRecursive(maze, wallX + 1, y1, x2, y2);
}
}
void generateMaze(int numRows, int numCols, int numSides) {
// Create maze cells
vector<vector<Cell*>> maze(numRows, vector<Cell*>(numCols));
for (int i = 0; i < numRows; i++) {
for (int j = 0; j < numCols; j++) {
maze[i][j] = new Cell(i, j, numSides);
}
}
// Generate maze
generateMazeRecursive(maze, 0, 0, numRows - 1, numCols - 1);
}
void solveMaze(vector<vector<Cell*>>& maze) {
stack<Cell*> stack;
Cell* start = maze[0][0];
Cell* end = maze[maze.size() - 1][maze[0].size() - 1];
stack.push(start);
start->visited = true;
while (!stack.empty()) {
Cell* current = stack.top();
if (current == end) {
break;
}
bool foundUnvisitedNeighbor = false;
for (int i = 0; i < current->numSides; i++) {
Cell* neighbor = current->neighbors[i];
if (!neighbor->visited) {
stack.push(neighbor);
neighbor->visited = true;
foundUnvisitedNeighbor = true;
break;
}
}
if (!foundUnvisitedNeighbor) {
stack.pop();
}
}
// Print solution
while (!stack.empty()) {
Cell* current = stack.top();
cout << "(" << current->x << ", " << current->y << ")" << endl;
stack.pop();
}
}
int main() {
srand(time(NULL));
generateMaze(10, 10, 6);
return 0;
}
```
阅读全文
相关推荐
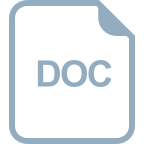
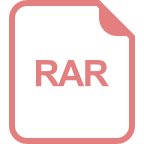

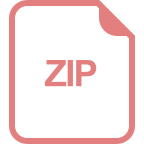
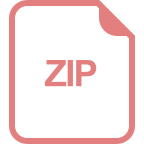
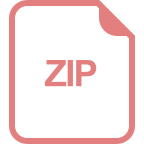
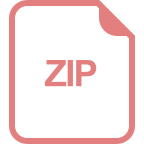
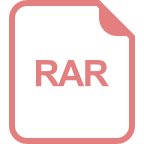
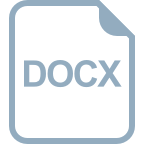
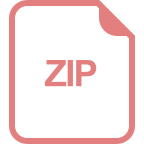
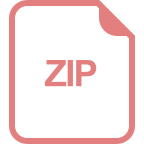