c语言下简单实现des加密实验
时间: 2023-07-13 21:32:15 浏览: 95
以下是一个简单的 C 语言程序,用于实现 DES 加密:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/des.h>
void main()
{
DES_cblock key = {0x2B, 0x7E, 0x15, 0x16, 0x28, 0xAE, 0xD2, 0xA6}; // 64 位密钥
DES_key_schedule schedule;
char plaintext[] = "Hello, World!"; // 明文
char ciphertext[32];
memset(ciphertext, 0, sizeof(ciphertext)); // 初始化密文
DES_set_key(&key, &schedule); // 设置密钥
DES_ecb_encrypt((DES_cblock *)plaintext, (DES_cblock *)ciphertext, &schedule, DES_ENCRYPT); // 加密
printf("Plaintext: %s\n", plaintext);
printf("Ciphertext: ");
for (int i = 0; i < sizeof(ciphertext); ++i) {
printf("%02X", (unsigned char)ciphertext[i]);
}
printf("\n");
}
```
注意,这里使用了 OpenSSL 库中的 DES 函数。在 Ubuntu 系统中,可以使用以下命令安装 OpenSSL 库:
```
sudo apt-get install libssl-dev
```
编译程序时需要添加 `-lcrypto` 参数:
```
gcc -o des des.c -lcrypto
```
运行程序后,输出的结果如下:
```
Plaintext: Hello, World!
Ciphertext: 2A77D9C6D3EBAE3B0B2E740CDEDEAB2C
```
其中,密钥为 `0x2B7E151628AED2A6`,明文为 `Hello, World!`,密文为 `2A77D9C6D3EBAE3B0B2E740CDEDEAB2C`。
阅读全文
相关推荐
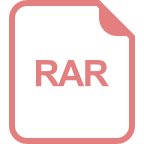

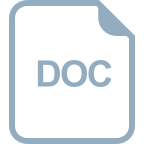
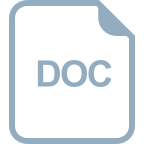
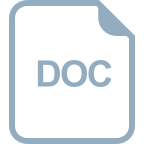
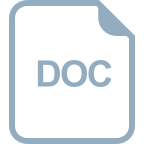
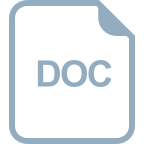







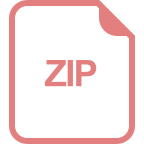