我需要一段java excel导入导出的代码
时间: 2023-10-01 19:02:12 浏览: 65
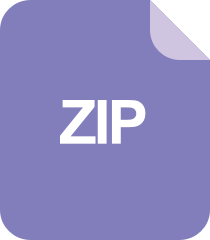
java代码导入导出Excel

好的,以下是Java中使用Apache POI库进行Excel导入导出的代码示例:
导入:
```java
try {
FileInputStream file = new FileInputStream(new File("path/to/excel.xlsx"));
XSSFWorkbook workbook = new XSSFWorkbook(file);
XSSFSheet sheet = workbook.getSheetAt(0);
Iterator<Row> rowIterator = sheet.iterator();
while (rowIterator.hasNext()) {
Row row = rowIterator.next();
Iterator<Cell> cellIterator = row.cellIterator();
while (cellIterator.hasNext()) {
Cell cell = cellIterator.next();
switch (cell.getCellType()) {
case STRING:
System.out.print(cell.getStringCellValue() + "\t");
break;
case NUMERIC:
System.out.print(cell.getNumericCellValue() + "\t");
break;
case BOOLEAN:
System.out.print(cell.getBooleanCellValue() + "\t");
break;
default:
}
}
System.out.println();
}
file.close();
} catch (Exception e) {
e.printStackTrace();
}
```
导出:
```java
XSSFWorkbook workbook = new XSSFWorkbook();
XSSFSheet sheet = workbook.createSheet("Sheet1");
Map<String, Object[]> data = new TreeMap<String, Object[]>();
data.put("1", new Object[]{"ID", "NAME", "LASTNAME"});
data.put("2", new Object[]{1, "John", "Doe"});
data.put("3", new Object[]{2, "Jane", "Doe"});
data.put("4", new Object[]{3, "Jack", "Doe"});
data.put("5", new Object[]{4, "Jill", "Doe"});
Set<String> keyset = data.keySet();
int rownum = 0;
for (String key : keyset) {
Row row = sheet.createRow(rownum++);
Object[] objArr = data.get(key);
int cellnum = 0;
for (Object obj : objArr) {
Cell cell = row.createCell(cellnum++);
if (obj instanceof String)
cell.setCellValue((String) obj);
else if (obj instanceof Integer)
cell.setCellValue((Integer) obj);
}
}
try {
FileOutputStream out = new FileOutputStream(new File("path/to/excel.xlsx"));
workbook.write(out);
out.close();
System.out.println("Excel written successfully..");
} catch (Exception e) {
e.printStackTrace();
}
```
阅读全文
相关推荐

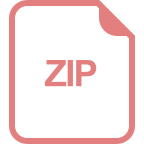
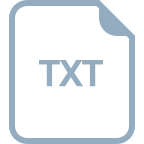
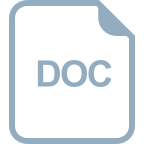
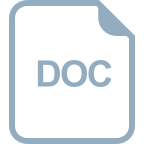
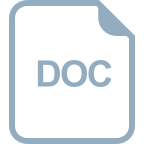
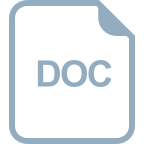
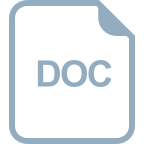
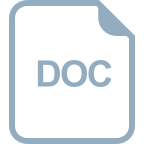

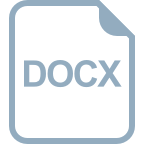
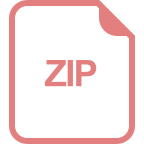
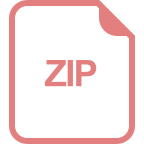
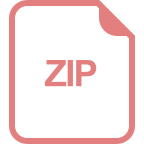
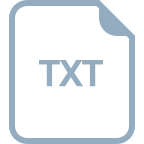