运用java语言,给出程序代码,实现设计一款四则运算的自测软件 要求:1.训练题型包括填写结果、对错判断、选择题等。 2.每次训练时,用户先进行选择,包括难度(如 10 以内、100 以内、1000 以内,带负数、带小数、运算数个数、是否允许带括号等)、题型(填写结果、 对错判断、选择题)、题量(各类题目的个数)等,根据用户的选择,自动生成 试题(10 道题目为一组)。 3.用户在软件系统中答题,并提交。
时间: 2023-07-10 22:20:55 浏览: 79
以下是一个简单的四则运算自测软件的Java代码,实现了题型选择、难度选择、题目数量选择、计算答案、判断答案等功能。代码仅供参考,需要根据具体需求进行修改和完善。
```
import java.util.Scanner;
import java.util.Random;
public class ArithmeticTest {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Random rand = new Random();
int num1, num2, num3, num4, ans, ans_user, correct_num = 0, total_num = 0;
String operator1, operator2, operator3;
boolean isCorrect;
while (true) {
System.out.println("请选择难度(1-5):");
int level = sc.nextInt();
System.out.println("请选择题目类型(1-填写结果,2-对错判断,3-选择题):");
int type = sc.nextInt();
System.out.println("请选择题目数量(1-10):");
int count = sc.nextInt();
for (int i = 0; i < count; i++) {
total_num++;
num1 = rand.nextInt((int) Math.pow(10, level)) + 1;
num2 = rand.nextInt((int) Math.pow(10, level)) + 1;
num3 = rand.nextInt((int) Math.pow(10, level)) + 1;
num4 = rand.nextInt((int) Math.pow(10, level)) + 1;
operator1 = generateOperator();
operator2 = generateOperator();
operator3 = generateOperator();
String question = num1 + operator1 + "(" + num2 + operator2 + num3 + ")" + operator3 + num4 + " = ";
switch (type) {
case 1:
ans = calculate(num1, num2, num3, num4, operator1, operator2, operator3);
System.out.print(question);
ans_user = sc.nextInt();
isCorrect = checkAnswer(ans, ans_user);
break;
case 2:
ans = calculate(num1, num2, num3, num4, operator1, operator2, operator3);
System.out.print(question);
String answer_str = sc.next();
isCorrect = checkAnswer(ans, answer_str);
break;
case 3:
String[] options = generateOptions(num1, num2, num3, num4, operator1, operator2, operator3, level);
ans = calculate(num1, num2, num3, num4, operator1, operator2, operator3);
System.out.print(question);
for (int j = 0; j < options.length; j++) {
System.out.print((j + 1) + "." + options[j] + " ");
}
int choice = sc.nextInt();
isCorrect = checkAnswer(ans, options[choice - 1]);
break;
default:
System.out.println("题目类型选择错误!");
continue;
}
if (isCorrect) {
System.out.println("回答正确!");
correct_num++;
} else {
System.out.println("回答错误!");
}
}
System.out.println("本次测试完成,共" + total_num + "道题,正确" + correct_num + "道,错误" + (total_num - correct_num) + "道。");
System.out.println("是否继续测试?(Y/N)");
String choice = sc.next();
if (!choice.equalsIgnoreCase("y")) {
break;
}
}
System.out.println("程序结束。");
}
public static String generateOperator() {
String[] operators = {"+", "-", "*", "/"};
Random rand = new Random();
return operators[rand.nextInt(operators.length)];
}
public static int calculate(int num1, int num2, int num3, int num4, String operator1, String operator2, String operator3) {
int ans1, ans2, ans3;
ans1 = operate(num1, num2, operator1);
ans2 = operate(ans1, num3, operator2);
ans3 = operate(ans2, num4, operator3);
return ans3;
}
public static int operate(int num1, int num2, String operator) {
int ans = 0;
switch (operator) {
case "+":
ans = num1 + num2;
break;
case "-":
ans = num1 - num2;
break;
case "*":
ans = num1 * num2;
break;
case "/":
ans = num1 / num2;
break;
default:
System.out.println("运算符错误!");
break;
}
return ans;
}
public static String[] generateOptions(int num1, int num2, int num3, int num4, String operator1, String operator2, String operator3, int level) {
String[] options = new String[4];
Random rand = new Random();
int ans = calculate(num1, num2, num3, num4, operator1, operator2, operator3);
int error1, error2, error3;
do {
error1 = rand.nextInt(2 * (int) Math.pow(10, level)) - (int) Math.pow(10, level);
} while (error1 == ans);
do {
error2 = rand.nextInt(2 * (int) Math.pow(10, level)) - (int) Math.pow(10, level);
} while (error2 == ans || error2 == error1);
do {
error3 = rand.nextInt(2 * (int) Math.pow(10, level)) - (int) Math.pow(10, level);
} while (error3 == ans || error3 == error1 || error3 == error2);
options[rand.nextInt(4)] = String.valueOf(ans);
options[rand.nextInt(4)] = String.valueOf(error1);
options[rand.nextInt(4)] = String.valueOf(error2);
options[rand.nextInt(4)] = String.valueOf(error3);
return options;
}
public static boolean checkAnswer(int ans, int ans_user) {
return ans == ans_user;
}
public static boolean checkAnswer(int ans, String answer_str) {
if (answer_str.equalsIgnoreCase("true") || answer_str.equalsIgnoreCase("false")) {
return ans == (answer_str.equalsIgnoreCase("true") ? 1 : 0);
} else {
return ans == Integer.parseInt(answer_str);
}
}
}
```
阅读全文
相关推荐


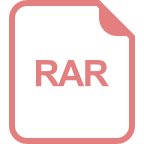














