使用Spring Cloud + Spring Boot构建微服务,建立分布式注册中心,进行分布式通信和设置分布式断路器例子
时间: 2023-08-23 16:23:31 浏览: 47
好的,下面我将为你提供一个使用Spring Cloud + Spring Boot构建微服务的例子,包括建立分布式注册中心、进行分布式通信和设置分布式断路器。
首先,我们需要建立一个分布式注册中心。可以使用Spring Cloud提供的Eureka Server来实现。在Spring Boot项目中添加如下依赖:
```xml
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-server</artifactId>
<version>2.2.5.RELEASE</version>
</dependency>
```
然后,创建一个启动类,并添加@EnableEurekaServer注解,如下所示:
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer;
@SpringBootApplication
@EnableEurekaServer
public class EurekaServerApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaServerApplication.class, args);
}
}
```
接下来,我们需要建立一个微服务提供者,可以使用Spring Boot提供的@RestController注解来实现。在Spring Boot项目中添加如下依赖:
```xml
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
<version>2.2.5.RELEASE</version>
</dependency>
```
然后,在启动类上添加@EnableDiscoveryClient注解,表示该微服务注册到Eureka Server中,如下所示:
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@EnableDiscoveryClient
@RestController
public class ServiceProviderApplication {
public static void main(String[] args) {
SpringApplication.run(ServiceProviderApplication.class, args);
}
@GetMapping("/hello")
public String hello() {
return "Hello, World!";
}
}
```
然后,我们需要建立一个微服务消费者,可以使用Spring Cloud提供的Feign来实现。在Spring Boot项目中添加如下依赖:
```xml
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
<version>2.2.5.RELEASE</version>
</dependency>
```
然后,在启动类上添加@EnableFeignClients注解,表示该微服务使用Feign进行微服务调用,如下所示:
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.openfeign.EnableFeignClients;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@EnableFeignClients
@RestController
public class ServiceConsumerApplication {
private final ServiceProviderClient serviceProviderClient;
public ServiceConsumerApplication(ServiceProviderClient serviceProviderClient) {
this.serviceProviderClient = serviceProviderClient;
}
public static void main(String[] args) {
SpringApplication.run(ServiceConsumerApplication.class, args);
}
@GetMapping("/hello")
public String hello() {
return serviceProviderClient.hello();
}
}
```
其中,ServiceProviderClient是一个Feign客户端,用于调用服务提供者。可以使用@FeignClient注解来定义该客户端,如下所示:
```java
import org.springframework.cloud.openfeign.FeignClient;
import org.springframework.web.bind.annotation.GetMapping;
@FeignClient("service-provider")
public interface ServiceProviderClient {
@GetMapping("/hello")
String hello();
}
```
最后,为了保证微服务的稳定性,我们可以设置分布式断路器。可以使用Spring Cloud提供的Hystrix来实现。在Spring Boot项目中添加如下依赖:
```xml
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-hystrix</artifactId>
<version>2.2.5.RELEASE</version>
</dependency>
```
然后,在Feign客户端的@FeignClient注解上添加fallback属性,指定回退类,如下所示:
```java
import org.springframework.cloud.openfeign.FeignClient;
import org.springframework.web.bind.annotation.GetMapping;
@FeignClient(value = "service-provider", fallback = ServiceProviderClientFallback.class)
public interface ServiceProviderClient {
@GetMapping("/hello")
String hello();
}
```
其中,ServiceProviderClientFallback是一个回退类,用于处理服务降级或熔断的情况,如下所示:
```java
import org.springframework.stereotype.Component;
@Component
public class ServiceProviderClientFallback implements ServiceProviderClient {
@Override
public String hello() {
return "Service Unavailable!";
}
}
```
以上就是使用Spring Cloud + Spring Boot构建微服务的示例,其中包括建立分布式注册中心、进行分布式通信和设置分布式断路器。希望能够对你有所帮助!
相关推荐
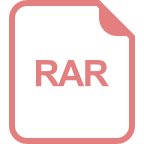
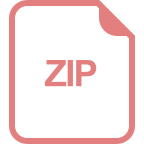
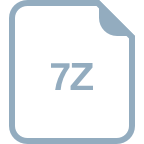
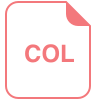
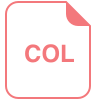
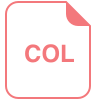
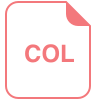
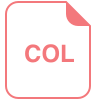









