Elasticsearch使用JestClient查询某一个字段的出现次数 Java代码实现
时间: 2024-05-13 08:16:03 浏览: 94
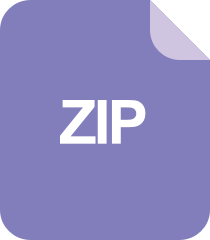
Elasticsearch-JestClient
可以使用Elasticsearch的聚合(Aggregation)功能来实现查询某一个字段的出现次数。以下是使用JestClient进行查询的Java代码示例:
```java
import io.searchbox.client.JestClient;
import io.searchbox.core.Search;
import io.searchbox.core.SearchResult;
import io.searchbox.params.Parameters;
import org.elasticsearch.index.query.QueryBuilders;
import org.elasticsearch.search.aggregations.AggregationBuilders;
import org.elasticsearch.search.aggregations.bucket.terms.Terms;
import org.elasticsearch.search.aggregations.metrics.sum.Sum;
import java.io.IOException;
public class ElasticsearchQuery {
public static void main(String[] args) throws IOException {
// 创建JestClient实例
JestClient jestClient = JestClientFactoryUtil.getJestClient();
// 构建查询语句
Search search = new Search.Builder("{\n" +
" \"query\": {\n" +
" \"match_all\": {}\n" +
" },\n" +
" \"aggs\": {\n" +
" \"count_by_field\": {\n" +
" \"terms\": {\n" +
" \"field\": \"field_name.keyword\"\n" + // 要查询的字段名
" }\n" +
" }\n" +
" }\n" +
"}\n")
.addIndex("index_name")
.addType("doc_type")
.setParameter(Parameters.SIZE, 0)
.build();
// 执行查询并获取结果
SearchResult result = jestClient.execute(search);
// 处理结果
Terms terms = result.getAggregations().getTermsAggregation("count_by_field");
for (Terms.Bucket bucket : terms.getBuckets()) {
String fieldValue = bucket.getKeyAsString();
long count = bucket.getDocCount();
System.out.println(fieldValue + "出现次数:" + count);
}
// 关闭JestClient实例
jestClient.shutdownClient();
}
}
```
在上面的代码示例中,我们使用了Elasticsearch的聚合功能来统计某一个字段的出现次数。具体来说,我们通过`AggregationBuilders.terms()`创建了一个Terms聚合,指定了要统计的字段名`field_name.keyword`,并将该聚合命名为`count_by_field`。在执行查询后,我们可以使用`result.getAggregations().getTermsAggregation("count_by_field")`方法获取该聚合的结果,然后遍历每一个Bucket,得到字段值和出现次数。
需要注意的是,由于我们只需要获取聚合结果而不需要查询的具体文档,因此可以将查询的`size`设置为0,从而减少查询的开销。另外,我们也可以使用`QueryBuilders`类来构建查询语句,以实现更复杂的查询需求。
阅读全文
相关推荐
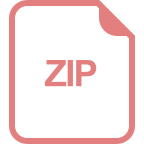
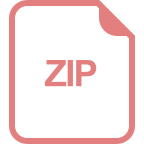















