如何统计一个String字符串中每个字符出现的个数
时间: 2023-08-31 07:27:42 浏览: 110
### 回答1:
您可以使用Java中的HashMap来统计一个String字符串中每个字符出现的个数。可以遍历这个String字符串,判断每一个字符是否存在于HashMap中,如果不存在,则将其添加到HashMap中,并将其出现的次数设置为1;如果存在,则将其出现的次数加1。最后,可以通过遍历HashMap中的所有元素,获取到每个字符出现的次数。
### 回答2:
要统计一个String字符串中每个字符出现的个数,可以使用HashMap来实现。
首先,将String字符串转化为字符数组,便于遍历每个字符。然后,创建一个HashMap对象,用于存储每个字符及其出现的次数。
接下来,遍历字符数组。对于每个字符,先判断该字符是否已经存在于HashMap中,如果不存在,则将该字符作为键,出现次数初始化为1并放入HashMap中;如果已存在,则将该字符对应的值加1。
最后,遍历完字符数组后,HashMap中存储了每个字符及其出现的次数。可以使用迭代器遍历HashMap,输出每个字符及其出现的次数。
以下是示例代码:
```java
import java.util.HashMap;
import java.util.Map;
public class CountCharacters {
public static void main(String[] args) {
String str = "aabbbccc";
Map<Character, Integer> charCountMap = new HashMap<>();
char[] charArray = str.toCharArray();
for (char c : charArray) {
if (charCountMap.containsKey(c)) {
int count = charCountMap.get(c);
charCountMap.put(c, count + 1);
} else {
charCountMap.put(c, 1);
}
}
System.out.println("字符出现的个数:");
for (Map.Entry<Character, Integer> entry : charCountMap.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
}
```
运行以上代码,输出结果为:
```
字符出现的个数:
a: 2
b: 3
c: 3
```
这样,就统计出了字符串中每个字符出现的个数。
### 回答3:
要统计一个String字符串中每个字符出现的个数,可以按照以下步骤进行:
1. 创建一个HashMap<Character, Integer>来存储每个字符和其出现的次数。
2. 遍历字符串中的每个字符。
3. 检查HashMap中是否已经存在该字符,如果存在,则将其对应的值加1;如果不存在,则将该字符作为键,值初始化为1,添加到HashMap中。
4. 遍历完所有字符后,HashMap中存储的字符和其出现次数就是所需的结果。
下面是一个Java代码示例:
```
public static HashMap<Character, Integer> countCharacters(String str){
HashMap<Character, Integer> charCountMap = new HashMap<>();
// 遍历字符串中的每个字符
for(int i = 0; i < str.length(); i++){
char ch = str.charAt(i);
// 判断HashMap中是否已经存在该字符
if(charCountMap.containsKey(ch)){
// 如果存在,则将其对应的值加1
charCountMap.put(ch, charCountMap.get(ch) + 1);
} else {
// 如果不存在,则将该字符作为键,值初始化为1,添加到HashMap中
charCountMap.put(ch, 1);
}
}
return charCountMap;
}
// 调用示例
public static void main(String[] args){
String str = "hello world";
HashMap<Character, Integer> result = countCharacters(str);
// 打印每个字符和其出现的次数
for(Character ch : result.keySet()){
System.out.println(ch + ": " + result.get(ch));
}
}
```
运行以上代码会输出如下结果:
```
h: 1
e: 1
l: 3
o: 2
: 1
w: 1
r: 1
d: 1
```
相关推荐
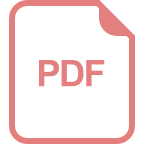
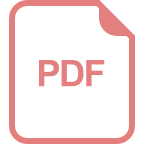
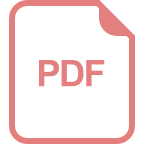
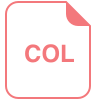
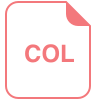
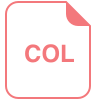
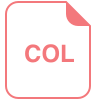










