用python编写一个图像全局线性变换的函数
时间: 2024-05-08 10:16:43 浏览: 10
下面是一个简单的 Python 代码示例,用于实现图像的全局线性变换:
```python
import cv2
import numpy as np
def global_linear_transform(image, alpha=1.0, beta=0.0):
"""Apply a global linear transformation to the given image."""
# Create a copy of the input image
result = image.copy()
# Compute the transformation matrix
matrix = np.array([[alpha, 0, beta],
[0, alpha, beta],
[0, 0, 1]])
# Apply the transformation matrix to the image
result = cv2.warpPerspective(result, matrix, result.shape[:2][::-1])
return result
```
这个函数接受一个图像作为输入,以及两个可选参数 alpha 和 beta,它们分别表示线性变换的缩放因子和偏移量。默认情况下,alpha 和 beta 都是 1.0 和 0.0,即不进行任何变换。
内部实现采用了 OpenCV 库的 warpPerspective 函数,该函数可以将图像应用于透视变换矩阵。我们使用 3x3 的变换矩阵来表示线性变换,其中前两行分别表示 x 和 y 坐标轴上的缩放和偏移,最后一行始终为 [0, 0, 1]。
使用该函数的示例:
```python
# Load an image
image = cv2.imread('example.jpg')
# Apply a global linear transformation with alpha=1.5 and beta=50
result = global_linear_transform(image, alpha=1.5, beta=50)
# Display the result
cv2.imshow('Result', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
相关推荐
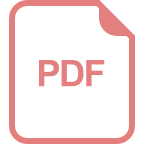
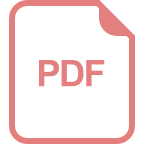
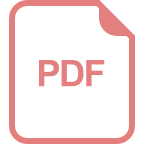

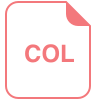
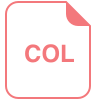

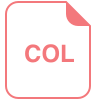









