写一段java反射获取注解的代码
时间: 2023-09-17 20:06:04 浏览: 119
### 回答1:
public void getAnnotation(Class clazz) {
Annotation[] annotations = clazz.getAnnotations();
for (Annotation annotation : annotations) {
System.out.println("注解名称:" + annotation.annotationType().getSimpleName());
}
}
### 回答2:
Java的反射机制可以用来获取类、方法、属性等的注解信息。下面是一个简单的示例代码,用于演示如何使用反射获取注解:
```java
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
@MyAnnotation(name = "TestClass")
public class TestClass {
@MyAnnotation(name = "TestMethod")
public void testMethod() {
System.out.println("This is a test method");
}
public static void main(String[] args) {
Class<TestClass> clazz = TestClass.class;
Annotation[] classAnnotations = clazz.getAnnotations();
for (Annotation annotation : classAnnotations) {
if (annotation instanceof MyAnnotation) {
MyAnnotation myAnnotation = (MyAnnotation) annotation;
System.out.println("Class Annotation Name: " + myAnnotation.name());
}
}
Method[] methods = clazz.getDeclaredMethods();
for (Method method : methods) {
Annotation[] methodAnnotations = method.getAnnotations();
for (Annotation annotation : methodAnnotations) {
if (annotation instanceof MyAnnotation) {
MyAnnotation myAnnotation = (MyAnnotation) annotation;
System.out.println("Method Annotation Name: " + myAnnotation.name());
}
}
}
}
}
@interface MyAnnotation {
String name();
}
```
在上面的代码中,我们首先定义了一个注解 `@MyAnnotation`,并将其分别应用到 `TestClass` 类和 `testMethod` 方法上。然后,通过反射获取 `TestClass` 类的注解和 `testMethod` 方法的注解,并将其输出到控制台上。
从输出结果来看,我们可以得到类注解的名称为 "TestClass",方法注解的名称为 "TestMethod"。
注意,以上只是一个简单的示例,实际使用反射获取注解时,可能需要结合具体的业务逻辑进行处理。
### 回答3:
反射是Java中强大的特性之一,可以在运行时动态获取类的信息,并且可以通过反射机制获取类、方法、字段等的注解信息。
下面是一个示例代码,用于演示如何使用Java反射获取注解:
```java
import java.lang.annotation.Annotation;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
// 定义一个注解
@interface MyAnnotation {
String value() default "";
}
// 定义一个类并添加注解
@MyAnnotation(value = "这是一个自定义注解")
class MyClass {
@MyAnnotation(value = "这是一个字段注解")
private String myField;
@MyAnnotation(value = "这是一个方法注解")
public void myMethod() {
System.out.println("Hello, Reflection!");
}
}
public class ReflectionDemo {
public static void main(String[] args) {
// 获取类注解
Class<MyClass> clazz = MyClass.class;
MyAnnotation classAnnotation = clazz.getAnnotation(MyAnnotation.class);
if (classAnnotation != null) {
System.out.println("类注解值:" + classAnnotation.value());
}
// 获取字段注解
try {
Field field = clazz.getDeclaredField("myField");
MyAnnotation fieldAnnotation = field.getAnnotation(MyAnnotation.class);
if (fieldAnnotation != null) {
System.out.println("字段注解值:" + fieldAnnotation.value());
}
} catch (NoSuchFieldException e) {
e.printStackTrace();
}
// 获取方法注解
try {
Method method = clazz.getMethod("myMethod");
MyAnnotation methodAnnotation = method.getAnnotation(MyAnnotation.class);
if (methodAnnotation != null) {
System.out.println("方法注解值:" + methodAnnotation.value());
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
}
}
}
```
在上面的代码中,我们首先定义了一个自定义注解`MyAnnotation`,并在`MyClass`类的类声明、字段和方法上添加了该注解。然后使用反射机制获取类注解、字段注解和方法注解,并从中获取注解的值打印输出。
运行上述代码,输出结果如下:
```
类注解值:这是一个自定义注解
字段注解值:这是一个字段注解
方法注解值:这是一个方法注解
```
以上就是使用Java反射获取注解的示例代码。通过这种方式,我们可以在运行时动态地获取类的注解信息,为程序的灵活性和扩展性提供了很大的便利。
阅读全文
相关推荐
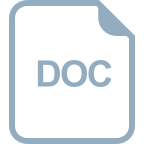
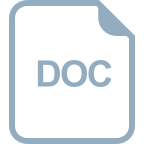
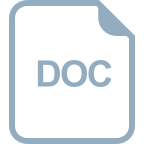
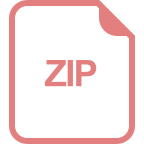
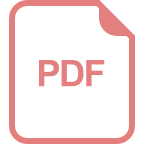
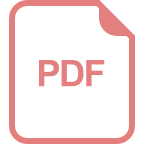
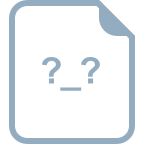
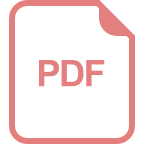
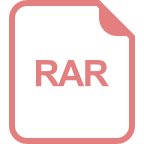
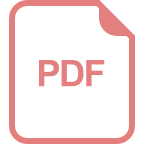
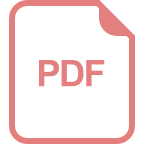
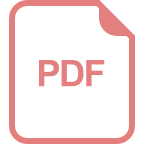
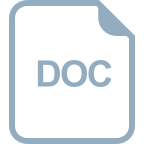
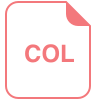
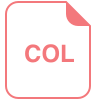
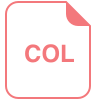


