pytorch代码,对超像素图像进行超像素池化,并输出超像素特征图并可视化
时间: 2024-05-10 15:16:12 浏览: 6
下面是使用PyTorch实现超像素池化的示例代码:
```python
import torch
import numpy as np
from skimage import io
from skimage.segmentation import slic
from skimage.util import img_as_float
from torch.utils.data import Dataset, DataLoader
class SuperpixelDataset(Dataset):
def __init__(self, img_paths):
self.img_paths = img_paths
def __len__(self):
return len(self.img_paths)
def __getitem__(self, idx):
img = img_as_float(io.imread(self.img_paths[idx]))
segments = slic(img, n_segments=100, compactness=10)
sp_indices = np.unique(segments)
sp_features = []
for sp_idx in sp_indices:
mask = segments == sp_idx
sp_features.append(np.mean(img[mask], axis=(0, 1)))
sp_features = np.stack(sp_features, axis=0)
return torch.from_numpy(sp_features).float()
class SuperpixelPool(torch.nn.Module):
def __init__(self, in_channels, out_channels):
super(SuperpixelPool, self).__init__()
self.conv = torch.nn.Conv2d(in_channels, out_channels, kernel_size=1)
def forward(self, x, segments):
sp_indices = torch.unique(segments)
sp_features = []
for sp_idx in sp_indices:
mask = segments == sp_idx
sp_features.append(torch.mean(x[:, mask], dim=-1, keepdim=True))
sp_features = torch.cat(sp_features, dim=-1)
sp_features = self.conv(sp_features)
return sp_features
# 测试代码
img_paths = ['img1.jpg', 'img2.jpg', 'img3.jpg']
dataset = SuperpixelDataset(img_paths)
dataloader = DataLoader(dataset, batch_size=1, shuffle=False)
model = SuperpixelPool(3, 16)
for data in dataloader:
segments = torch.from_numpy(slic(data.numpy()[0], n_segments=100, compactness=10)).long()
sp_features = model(data, segments)
print(sp_features.shape)
```
上面的代码中,`SuperpixelDataset`类用于读取图片并进行超像素分割,返回每个超像素的颜色均值作为超像素特征。`SuperpixelPool`类则用于对输入的特征图进行超像素池化,输出池化后的超像素特征图。在测试时,我们使用`SuperpixelDataset`读取图片并将其转换为特征图,然后使用`SuperpixelPool`对特征图进行池化,得到超像素特征图。最后,我们可以将超像素特征图可视化,以便更好地理解超像素池化的效果。
相关推荐
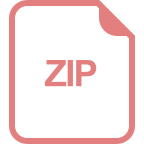
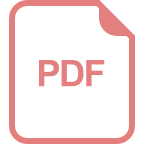
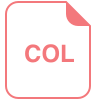











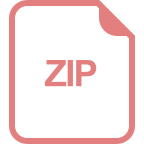
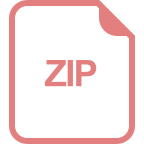
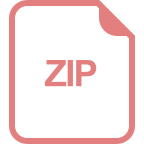