{ int count = 0; for (int i = 0; i < enrollments.size(); ++i) { if (enrollments[i].courseId == courseId) { count++; } } return count; } void queryEnrollmentCount() { int courseId; cout << "请输入要查询的课程编号:"; cin >> courseId; Course* course = NULL; for (int i = 0; i < courses.size(); ++i) { if (courses[i].id == courseId) { course = &courses[i]; break; } } if (course == NULL) { cout << "课程不存在!" << endl; return; } int count = countEnrollment(courseId); cout << "课程 " << course->name << " 的选课人数为:" << count << endl; } int calculateCredits(int studentId, int year) { int totalCredits = 0; for (int i = 0; i < enrollments.size(); ++i) { if (enrollments[i].studentId == studentId && enrollments[i].year == year && enrollments[i].grade >= 60) { Course* course = NULL; for (int j = 0; j < courses.size(); ++j) { if (courses[j].id == enrollments[i].courseId) { course = &courses[j]; break; } } if (course != NULL) { totalCredits += course->credit; } } } return totalCredits; }
时间: 2024-02-14 11:26:17 浏览: 130
这段代码包含了三个函数:`queryEnrollmentCount`、`countEnrollment`和`calculateCredits`。
`queryEnrollmentCount`函数用于查询特定课程的选课人数。它首先要求用户输入要查询的课程编号,然后在`courses`列表中查找匹配的课程。如果找到了匹配的课程,就调用`countEnrollment`函数计算该课程的选课人数,并输出结果。
`countEnrollment`函数用于计算与给定课程ID匹配的选课人数。它通过遍历`enrollments`列表,对每个注册的课程ID进行比较,如果匹配则计数加一。
`calculateCredits`函数用于计算特定学生在特定年份所修学分总数。它遍历`enrollments`列表,对每个满足条件的选课记录进行判断:学生ID与给定的学生ID相同、年份与给定的年份相同并且成绩大于等于60。对于满足条件的课程,在`courses`列表中查找匹配的课程,并将该课程的学分累加到总学分中。
请注意,以上代码只是函数的框架,实际的数据结构和具体实现可能需要根据实际情况进行适当调整。
相关问题
enrollments[i].courseId == courseId
这是一个IT类的问题,我可以直接回答。
该语句是一个条件判断语句,用于检查一个数组中的enrollments对象的courseId属性是否等于给定的courseId。如果相等,则条件为真,返回true;否则,条件为假,返回false。这个语句通常用于筛选出特定courseId的enrollments对象。
Develop a python class named Enroll to simulate the course registration process. It should include (1) class attribute: enrollmentcount which counts the total number of enrollments, and initiates to 0 (2) static method named buildcourselist(filepath) that creates a course list from a text file that has such text Id Title Credit CSCI120 Elements of Computing 4 INFO230 Intro to Web Design 3 CSCI230 System Architecture 3 (the column separator has 2+ spaces, 列分隔用2+空格, file attached) The method should return a python list of Course objects (hint: A. open file for read, new a list, read line by line, for each line, create a Course object and insert into the list. Ignore the first line. B. a static method is also called class method, does not take the self argument ) (3) static method named buildstudentlist(filepath) that creates a Student list from a text file that has such text Id Name D10000000 Zhang Hong D10000001 Chen Huayue The method should return a python list of Student objects
Sure, here's an example implementation of the Enroll class in Python:
```python
class Course:
def __init__(self, course_id, title, credit):
self.course_id = course_id
self.title = title
self.credit = credit
class Student:
def __init__(self, student_id, name):
self.student_id = student_id
self.name = name
class Enroll:
enrollmentcount = 0
@staticmethod
def buildcourselist(filepath):
course_list = []
with open(filepath, 'r') as f:
lines = f.readlines()[1:] # Ignore header line
for line in lines:
data = line.strip().split(' ')
course = Course(data[0], data[1], int(data[2]))
course_list.append(course)
return course_list
@staticmethod
def buildstudentlist(filepath):
student_list = []
with open(filepath, 'r') as f:
lines = f.readlines()
for line in lines:
data = line.strip().split(' ')
student = Student(data[0], data[1])
student_list.append(student)
return student_list
```
This implementation defines the `Enroll` class with a class attribute `enrollmentcount` that counts the total number of enrollments. It also includes two static methods: `buildcourselist` and `buildstudentlist`, which read data from text files and create lists of `Course` and `Student` objects respectively.
To use this implementation, you can call the static methods on the `Enroll` class, for example:
```python
courses = Enroll.buildcourselist('courses.txt')
students = Enroll.buildstudentlist('students.txt')
```
Assuming that `courses.txt` and `students.txt` are text files in the format described in the question, this code will create lists of `Course` and `Student` objects that you can use for simulating the course registration process.
阅读全文
相关推荐
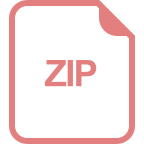
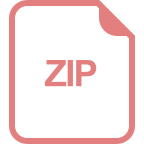
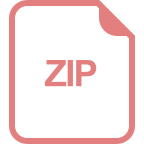













