通过/sys/class/pwm/pwmchip0/enable文件控制pwm使能的demoC语言函数
时间: 2023-09-05 12:08:40 浏览: 217
下面是一个简单的控制PWM使能的demo C语言函数:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
void enable_pwm(int pwm_num) {
char enable_path[50];
sprintf(enable_path, "/sys/class/pwm/pwmchip0/pwm%d/enable", pwm_num);
int fd = open(enable_path, O_WRONLY);
if (fd == -1) {
perror("Failed to open enable file");
exit(1);
}
write(fd, "1", 1);
close(fd);
}
```
该函数的参数`pwm_num`表示要控制的PWM通道号。函数内部将该通道对应的使能文件路径构造出来,然后通过`open`函数打开该文件,再通过`write`函数将字符“1”写入该文件,从而完成PWM使能。最后,通过`close`函数关闭文件描述符。
相关问题
读取/sys/class/pwm/pwmchip0/enable的内容demoC语言函数
可以使用以下的demo C语言函数读取`/sys/class/pwm/pwmchip0/enable`的内容:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_BUF 64
int read_pwm_enable()
{
FILE* fp;
char buf[MAX_BUF];
int enable;
fp = fopen("/sys/class/pwm/pwmchip0/enable", "r");
if (fp == NULL) {
printf("Error: Failed to open file /sys/class/pwm/pwmchip0/enable.\n");
return -1;
}
fgets(buf, MAX_BUF, fp);
enable = atoi(buf);
fclose(fp);
return enable;
}
```
这个函数使用了标准的C库函数`fopen()`、`fgets()`和`fclose()`,以及`stdlib.h`中的`atoi()`函数。函数返回`/sys/class/pwm/pwmchip0/enable`文件中的整数值。如果函数无法打开文件或者读取失败,函数返回-1。
读取/sys/class/pwm/pwmchip0/polarity的内容demoC语言函数
可以使用以下的C语言函数来读取/sys/class/pwm/pwmchip0/polarity的内容:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BUF 256
int main() {
FILE *fp;
char path[MAX_BUF];
char buf[MAX_BUF];
// 构建文件路径
snprintf(path, sizeof(path), "/sys/class/pwm/pwmchip0/polarity");
// 打开文件
fp = fopen(path, "r");
if (fp == NULL) {
printf("Failed to open file: %s\n", path);
return 1;
}
// 读取文件内容
fgets(buf, sizeof(buf), fp);
// 关闭文件
fclose(fp);
// 输出文件内容
printf("Content of %s: %s", path, buf);
return 0;
}
```
该程序通过构建文件路径,打开并读取文件内容,最后输出文件内容。需要注意的是,该程序只能读取文件内容,不能对文件进行修改。
阅读全文
相关推荐
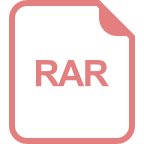
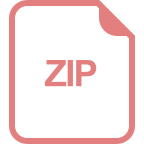
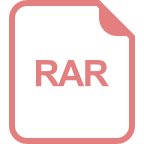





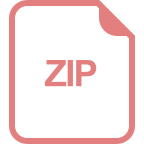
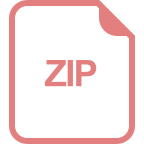
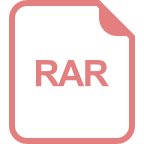
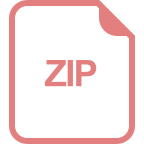
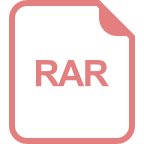
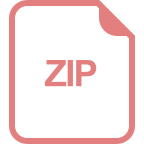
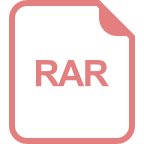
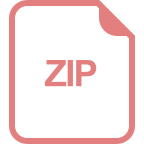
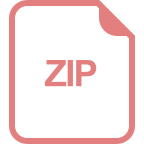