PID_State = PID_Increament(PID_Calibration, PID_State);
时间: 2024-03-29 09:25:05 浏览: 19
这行代码是一个PID控制器的实现,用于计算当前时刻的控制量。其中,PID_Calibration是PID控制器的参数,包括比例系数、积分系数和微分系数等;PID_State是当前的控制状态,包括当前误差、上一个误差和累计误差等。PID_Increament是PID控制器的计算函数,它会根据PID_Calibration和PID_State计算出当前的控制量,并返回给PID_State,供下一次计算使用。
相关问题
优化一下代码static void fill_file_chunk(const char *path, int *number, int level) { DIR *dir = NULL; struct dirent *entry = NULL; int i = 0; ++level; if(level > 3) return; dir = opendir(path); if(dir == NULL) return; while((entry = readdir(dir)) != NULL) { if(strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0) continue; if(entry->d_type == 4 && level == 1){ if(check_dir_name(entry->d_name) == 0) continue; char buffer[MAX_PATH]; sprintf(buffer, "%s/%s", path, entry->d_name); fill_file_chunk(buffer, number, level); } if(entry->d_type == 4 && level == 2){ if(check_dir_name(entry->d_name) == 0) continue; char buffer[MAX_PATH]; sprintf(buffer, "%s/%s", path, entry->d_name); fill_file_chunk(buffer, number, level); } if(entry->d_type == 8 && level ==3){ if(check_file_name(entry->d_name) == 0) continue; if(*number == max_file_chunk){ file_chunks = (struct FileChunk *)realloc(file_chunks, (max_file_chunk + FILE_CHUNK_INCREAMENT) * sizeof(struct FileChunk)); if(file_chunks == NULL) break; max_file_chunk += FILE_CHUNK_INCREAMENT; } char buffer[MAX_PATH]; sprintf(buffer, "%s/%s", path, entry->d_name); i = *number; strcpy(file_chunks[i].file_name, entry->d_name); sprintf(file_chunks[i].file_path, buffer); file_chunks[i].file_size = get_file_size(buffer); *number = i + 1; } else continue; } closedir(dir); return; }
Here are some suggested optimizations for the given code:
1. Use switch case instead of if-else: In the current code, multiple if-else statements are being used to check for different conditions. This can be optimized by using switch case instead, which can improve readability and maintainability of the code.
2. Use early return to reduce nested if-else: In the current code, multiple if-else statements are nested inside a while loop, which can make the code difficult to read and understand. By using early return, we can reduce the level of nesting and make the code more readable.
3. Avoid redundant code: In the current code, there are multiple instances where the same block of code is being repeated. This can be optimized by refactoring the code and avoiding the duplication of code.
Here is the optimized code:
```
static void fill_file_chunk(const char *path, int *number, int level) {
DIR *dir = opendir(path);
if(dir == NULL) {
return;
}
++level;
if(level > 3) {
closedir(dir);
return;
}
struct dirent *entry;
while((entry = readdir(dir)) != NULL) {
switch(entry->d_type) {
case DT_DIR:
if(level == 1 || level == 2) {
if(check_dir_name(entry->d_name) == 0) {
continue;
}
char buffer[MAX_PATH];
sprintf(buffer, "%s/%s", path, entry->d_name);
fill_file_chunk(buffer, number, level);
}
break;
case DT_REG:
if(level == 3) {
if(check_file_name(entry->d_name) == 0) {
continue;
}
if(*number == max_file_chunk) {
file_chunks = (struct FileChunk *)realloc(file_chunks, (max_file_chunk + FILE_CHUNK_INCREAMENT) * sizeof(struct FileChunk));
if(file_chunks == NULL) {
break;
}
max_file_chunk += FILE_CHUNK_INCREAMENT;
}
char buffer[MAX_PATH];
sprintf(buffer, "%s/%s", path, entry->d_name);
int i = *number;
strcpy(file_chunks[i].file_name, entry->d_name);
sprintf(file_chunks[i].file_path, buffer);
file_chunks[i].file_size = get_file_size(buffer);
*number = i + 1;
}
break;
default:
break;
}
}
closedir(dir);
}
```
This optimized code uses switch case instead of if-else statements, reduces the level of nesting by using early return, and avoids redundant code by refactoring the code.
check the manual that corresponds to your MySQL server version for the right syntax to use near 'auto_increament primary key
The correct syntax for creating an auto-increment primary key column in MySQL is as follows:
```sql
CREATE TABLE table_name (
column_name datatype AUTO_INCREMENT PRIMARY KEY,
...
);
```
Make sure to replace `table_name` with the actual name of your table and `column_name` with the name you want to give to the column. Additionally, specify the appropriate data type for your column.
For example, if you have a table called "users" and you want to create an auto-increment primary key column named "id", you can use the following SQL statement:
```sql
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
...
);
```
Remember that the specific syntax may vary depending on your MySQL server version, so always refer to the manual corresponding to your server version for accurate information.
相关推荐
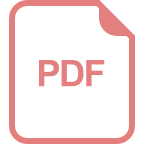
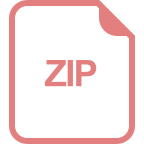
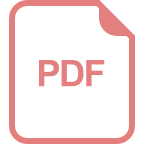


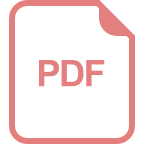
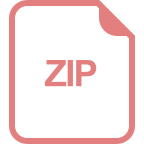
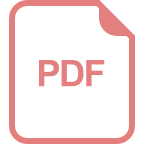
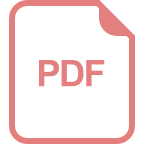
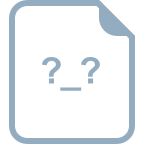
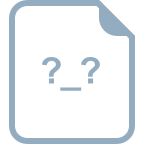
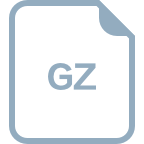
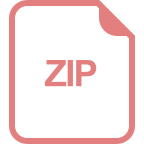