import json import os #指定原始数据集和目标路径 json_folder_path = "D:/python/pycharm/pycharm_date/Oracle/OracleCS/ce_shi_label" txt_folder_path = "D:/python/pycharm/pycharm_date/Oracle/OracleCS/labels" #获取所有json文件的路径 json_files = [os.path.join(json_folder_path, f) for f in os.listdir(json_folder_path) if f.endswith('.json')] for json_file in json_files:#打开json文件 with open(json_file, 'r', encoding='utf-8') as f:data = json.load(f) image_name = data["img_name"] + ".jpg" label_name = data["img_name"] boxes = data["ann"] #将每个矩形框转换为txt格式 txt_data = "" txt_line_1 = f"{label_name}\n" for box in boxes: x1, y1,x2, y2,class_id = str(box[0]), str(box[1]), str(box[2]),str(box[3]),str(box[4]) txt_line =f"{x1} {y1} {x2} {y2} {class_id}\n" txt_data += txt_line #写入txt文件 txt_file = os.path.join(txt_folder_path, image_name.replace(".jpg", ".txt")) with open(txt_file, 'w', encoding='utf-8') as f:f.write(txt_line_1 + txt_data)将其中json文件的名称统一放在一一个新的txt文件中
时间: 2023-12-14 20:36:52 浏览: 126
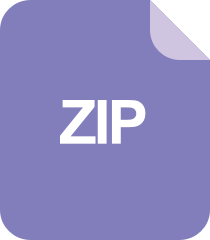
pyon:使用JSON的Python方式-具有本机对象:hammer_and_wrench:和路径支持:file_folder:
可以在循环外部先创建一个空的列表,然后在循环中每次将json文件的名称添加到列表中。循环结束后再将列表中的元素写入到一个新的txt文件中即可。
示例代码如下:
```python
import json
import os
# 指定原始数据集和目标路径
json_folder_path = "D:/python/pycharm/pycharm_date/Oracle/OracleCS/ce_shi_label"
txt_folder_path = "D:/python/pycharm/pycharm_date/Oracle/OracleCS/labels"
# 获取所有json文件的路径
json_files = [os.path.join(json_folder_path, f) for f in os.listdir(json_folder_path) if f.endswith('.json')]
# 创建空的列表
json_names = []
for json_file in json_files:
# 打开json文件
with open(json_file, 'r', encoding='utf-8') as f:
data = json.load(f)
image_name = data["img_name"] + ".jpg"
label_name = data["img_name"]
boxes = data["ann"]
# 将json文件名称添加到列表中
json_names.append(label_name)
# 将每个矩形框转换为txt格式
txt_data = ""
txt_line_1 = f"{label_name}\n"
for box in boxes:
x1, y1,x2, y2,class_id = str(box[0]), str(box[1]), str(box[2]),str(box[3]),str(box[4])
txt_line =f"{x1} {y1} {x2} {y2} {class_id}\n"
txt_data += txt_line
# 写入txt文件
txt_file = os.path.join(txt_folder_path, image_name.replace(".jpg", ".txt"))
with open(txt_file, 'w', encoding='utf-8') as f:
f.write(txt_line_1 + txt_data)
# 将json文件名称写入新的txt文件中
json_names_file = os.path.join(txt_folder_path, "json_names.txt")
with open(json_names_file, 'w', encoding='utf-8') as f:
for name in json_names:
f.write(name + "\n")
```
这样,就可以将所有json文件的名称统一放在一个新的txt文件中了。
阅读全文
相关推荐
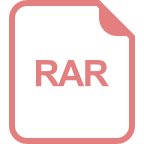
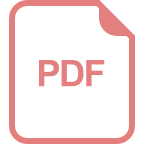
















