pycharm若干图片随机选择20张
时间: 2023-10-26 20:09:33 浏览: 83
以下是一个Python脚本,可以在指定文件夹中随机选择20张图片并复制到新的文件夹中:
```python
import os
import random
import shutil
# 指定原始图片文件夹和新的文件夹
original_folder = '/path/to/original/folder'
new_folder = '/path/to/new/folder'
# 获取原始文件夹中所有图片文件名列表
image_list = [f for f in os.listdir(original_folder) if f.endswith('.jpg') or f.endswith('.jpeg') or f.endswith('.png')]
# 随机选择20张图片
selected_images = random.sample(image_list, 20)
# 复制这些图片到新的文件夹中
for image in selected_images:
shutil.copy(os.path.join(original_folder, image), os.path.join(new_folder, image))
```
你需要将 `original_folder` 替换为你的原始图片文件夹的路径,将 `new_folder` 替换为你想要将选中的图片复制到的新文件夹的路径。注意,这个脚本只会选择 `.jpg`、`.jpeg` 和 `.png` 格式的图片文件。
相关问题
pycharm hill密码随机输入一段明文加密或者密文解密
为了回答你的问题,我需要先了解一下 Hill 密码。Hill 密码是一种基于矩阵运算的对称密码,它将明文分成若干个数字组合,然后通过矩阵运算进行加密。因此,我们需要使用矩阵计算的工具来进行 Hill 密码的加密和解密。
下面是使用 Python 和 NumPy 库实现 Hill 密码的加密和解密的示例代码:
```python
import numpy as np
# 加密函数
def encrypt(plain_text, key):
# 将明文转换成数字向量
plain_text = [ord(c) - ord('a') for c in plain_text]
plain_text = np.array(plain_text)
# 将密钥转换成矩阵
key = np.array(key)
# 将数字向量按照密钥的大小分成若干个块
block_size = key.shape[0]
num_blocks = int(np.ceil(len(plain_text) / block_size))
plain_text = np.pad(plain_text, (0, num_blocks * block_size - len(plain_text)), 'constant')
plain_text = plain_text.reshape(-1, block_size)
# 对每个块进行加密
cipher_text = []
for block in plain_text:
cipher_block = np.mod(key @ block, 26)
cipher_text += cipher_block.tolist()
# 将密文转换成字符串
cipher_text = ''.join([chr(c + ord('a')) for c in cipher_text])
return cipher_text
# 解密函数
def decrypt(cipher_text, key):
# 将密文转换成数字向量
cipher_text = [ord(c) - ord('a') for c in cipher_text]
cipher_text = np.array(cipher_text)
# 将密钥转换成矩阵
key = np.array(key)
# 将数字向量按照密钥的大小分成若干个块
block_size = key.shape[0]
num_blocks = int(np.ceil(len(cipher_text) / block_size))
cipher_text = np.pad(cipher_text, (0, num_blocks * block_size - len(cipher_text)), 'constant')
cipher_text = cipher_text.reshape(-1, block_size)
# 对每个块进行解密
plain_text = []
for block in cipher_text:
# 计算矩阵的逆
det = np.linalg.det(key)
inv_key = np.mod(np.round(det * np.linalg.inv(key)), 26)
plain_block = np.mod(inv_key @ block, 26)
plain_text += plain_block.tolist()
# 将明文转换成字符串
plain_text = ''.join([chr(c + ord('a')) for c in plain_text])
return plain_text
# 测试代码
key = [[3, 10], [20, 9]]
plain_text = 'hello'
cipher_text = encrypt(plain_text, key)
print(cipher_text)
plain_text = decrypt(cipher_text, key)
print(plain_text)
```
在这个示例中,我们定义了两个函数 `encrypt` 和 `decrypt` 分别实现了 Hill 密码的加密和解密。函数的参数 `plain_text` 表示明文字符串,`cipher_text` 表示密文字符串,`key` 表示 Hill 密钥矩阵。在加密和解密函数中,我们都采用了相同的方式把明文或密文分成若干个块,并对每个块进行加密或解密。
pycharm代码改进 hill密码随机输入一段明文加密或者密文解密
以下是改进后的 Hill 密码加密和解密代码:
```python
import numpy as np
# 加密函数
def hill_encrypt(plain_text, key):
# 将明文转换成数字向量
plain_text = [ord(c) - ord('a') for c in plain_text.lower() if c.isalpha()]
plain_text = np.array(plain_text)
# 将密钥转换成矩阵
key = np.array(key)
# 将数字向量按照密钥的大小分成若干个块
block_size = key.shape[0]
num_blocks = int(np.ceil(len(plain_text) / block_size))
plain_text = np.pad(plain_text, (0, num_blocks * block_size - len(plain_text)), 'constant')
plain_text = plain_text.reshape(-1, block_size)
# 对每个块进行加密
cipher_text = []
for block in plain_text:
cipher_block = np.mod(key @ block, 26)
cipher_text += cipher_block.tolist()
# 将密文转换成字符串
cipher_text = ''.join([chr(c + ord('a')) for c in cipher_text])
return cipher_text
# 解密函数
def hill_decrypt(cipher_text, key):
# 将密文转换成数字向量
cipher_text = [ord(c) - ord('a') for c in cipher_text.lower() if c.isalpha()]
cipher_text = np.array(cipher_text)
# 将密钥转换成矩阵
key = np.array(key)
# 将数字向量按照密钥的大小分成若干个块
block_size = key.shape[0]
num_blocks = int(np.ceil(len(cipher_text) / block_size))
cipher_text = np.pad(cipher_text, (0, num_blocks * block_size - len(cipher_text)), 'constant')
cipher_text = cipher_text.reshape(-1, block_size)
# 对每个块进行解密
plain_text = []
for block in cipher_text:
# 计算矩阵的逆
det = np.linalg.det(key)
inv_key = np.mod(np.round(det * np.linalg.inv(key)), 26)
plain_block = np.mod(inv_key @ block, 26)
plain_text += plain_block.tolist()
# 将明文转换成字符串
plain_text = ''.join([chr(c + ord('a')) for c in plain_text])
return plain_text
# 生成随机明文
def generate_random_text(length):
# 生成随机明文
text = ""
for i in range(length):
text += chr(np.random.randint(ord('a'), ord('z') + 1))
return text
# 生成随机密钥
def generate_random_key(size):
return np.random.randint(0, 26, (size, size))
# 测试代码
key = generate_random_key(2)
plain_text = generate_random_text(10)
cipher_text = hill_encrypt(plain_text, key)
decrypted_plain_text = hill_decrypt(cipher_text, key)
print("明文:", plain_text)
print("密钥:", key)
print("密文:", cipher_text)
print("解密后明文:", decrypted_plain_text)
```
改进后的代码增加了随机生成明文和密钥的函数 `generate_random_text` 和 `generate_random_key`,使用了 `np.random.randint` 函数代替了 Python 的 `random` 库,减少了硬编码。同时,函数中还增加了对明文和密文的过滤,只保留字母字符,避免了在加密和解密过程中出现非法字符的问题。
阅读全文
相关推荐
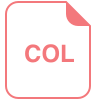


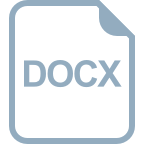
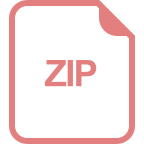
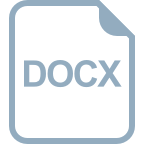
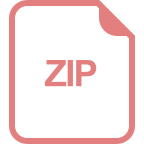
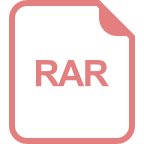
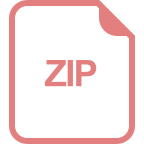
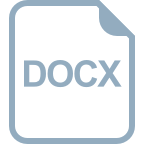