tensorflow中tf.nn.sigmoid有什么作用?它的用法是什么
时间: 2024-03-18 18:42:02 浏览: 112
tf.nn.sigmoid是一个激活函数,它的作用是将一个数值压缩到0和1之间。它的公式为:sigmoid(x) = 1 / (1 + exp(-x))。在神经网络中,sigmoid函数常用于解决二元分类问题,因为它能将输出值映射到0和1之间,可以看作是一个概率值,表示该样本属于正类的概率。
在tensorflow中,tf.nn.sigmoid函数的用法如下:
```python
import tensorflow as tf
x = tf.constant([1.0, 2.0, 3.0])
y = tf.nn.sigmoid(x)
with tf.Session() as sess:
print(sess.run(y))
```
输出结果为:
```
[0.7310586 0.880797 0.95257413]
```
这个例子中,我们将x输入到sigmoid函数中,得到一个输出y,表示x经过sigmoid函数的压缩后的结果。其中,tf.constant创建了一个常量张量x,tf.nn.sigmoid函数对x进行了计算,并将结果存储在y中,最后用Session来运行y,得到输出结果。
相关问题
import tensorflow as tf a = tf.constant([[1,2,3,4,5], [2,3,4,5,6]], dtype=tf.float32) indices = tf.constant([[1, 0, 1, 0, 0], [0, 1, 0, 0, 0]], dtype = tf.int32) result = tf.where(tf.cast(indices, dtype=tf.bool), tf.nn.sigmoid(a), a) with tf.Session() as sess: print(sess.run(result))
这段代码使用 TensorFlow 定义了一个二维张量 `a` 和一个与之对应的二维索引张量 `indices`。然后,使用 TensorFlow 提供的 `tf.where` 方法,将 `a` 张量中对应 `indices` 张量中为 1 的位置的值应用 sigmoid 函数,其他位置的值不变,最终生成一个新的张量 `result`。
具体来说,`tf.cast(indices, dtype=tf.bool)` 将 `indices` 张量中的值转换为布尔类型,即将 1 转换为 True,将 0 转换为 False。`tf.where` 使用这个布尔类型的掩码来决定是否应用 sigmoid 函数。对于 `indices` 张量中为 1 的位置,`tf.where` 返回 `tf.nn.sigmoid(a)`,即对应位置的 `a` 张量的值应用 sigmoid 函数;对于 `indices` 张量中为 0 的位置,`tf.where` 返回 `a` 张量中对应的原始值。
最后,使用 TensorFlow Session 执行这个计算图,并打印 `result` 张量的值。
用tensorflow的layers.Layer模块改写 class SpatialAttention(nn.Module): def init(self): super(SpatialAttention, self).init() self.conv1 = nn.Conv2d(2, 1, 3, padding = 1, bias=False) self.sigmoid = nn.Sigmoid() def forward(self, x): avg_out = torch.mean(x, dim = 1, keepdim = True) max_out, _ = torch.max(x, dim = 1, keepdim = True) x = torch.cat([avg_out, max_out], dim = 1) x = self.conv1(x) return self.sigmoid(x)
以下是使用TensorFlow的layers.Layer模块改写的代码:
```python
import tensorflow as tf
class SpatialAttention(tf.keras.layers.Layer):
def __init__(self):
super(SpatialAttention, self).__init__()
self.conv1 = tf.keras.layers.Conv2D(1, 3, padding='same', use_bias=False)
self.sigmoid = tf.keras.layers.Activation('sigmoid')
def call(self, inputs):
avg_out = tf.reduce_mean(inputs, axis=1, keepdims=True)
max_out = tf.reduce_max(inputs, axis=1, keepdims=True)
x = tf.concat([avg_out, max_out], axis=1)
x = self.conv1(x)
return self.sigmoid(x)
```
在TensorFlow的layers.Layer模块中,我们使用`__init__()`方法来初始化层的参数,使用`call()`方法来定义层的前向传播逻辑。`Conv2D`和`Activation`分别对应PyTorch中的`nn.Conv2d`和`nn.Sigmoid`。`reduce_mean`和`reduce_max`分别对应PyTorch中的`torch.mean`和`torch.max`。`concat`用于在给定维度上连接不同的张量。
阅读全文
相关推荐
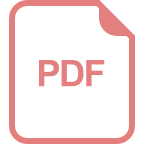
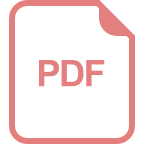
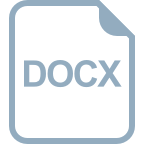

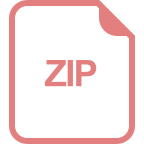
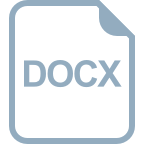
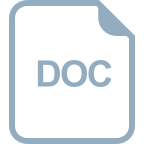
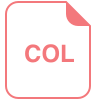
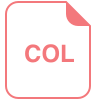
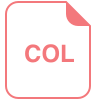
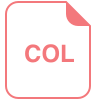
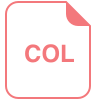
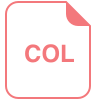

