java上传excel表格,校验以后,将错误的原因写入到excel中,支持下载
时间: 2023-11-12 19:03:39 浏览: 35
答案:
您可以使用Apache POI库来读取和写入Excel文件,使用Java代码来实现该功能。您需要编写代码来读取表格中的数据,进行验证,并将错误信息写入Excel表格中。下面是一个简单示例代码,可以帮助您开始实现这个功能:
```
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFCell;
import org.apache.poi.xssf.usermodel.XSSFRow;
import org.apache.poi.xssf.usermodel.XSSFSheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.util.ArrayList;
import java.util.List;
public class ExcelValidator {
public static void main(String[] args) {
String inputFile = "input.xlsx";
String outputFile = "output.xlsx";
try {
FileInputStream inputStream = new FileInputStream(inputFile);
XSSFWorkbook workbook = new XSSFWorkbook(inputStream);
XSSFSheet sheet = workbook.getSheetAt(0);
int rowNum = sheet.getLastRowNum() + 1;
List<String> errors = new ArrayList<>();
// Validate the data in the sheet
for (int i = 1; i < rowNum; i++) {
XSSFRow row = sheet.getRow(i);
// Check if the first cell is not empty
XSSFCell firstCell = row.getCell(0);
if (firstCell == null || firstCell.getCellType() != CellType.STRING) {
errors.add(String.format("Row %d: First cell is empty or not a string", i + 1));
// Skip the rest of the validation
continue;
}
// Check if the second cell contains a valid number
XSSFCell secondCell = row.getCell(1);
if (secondCell == null || secondCell.getCellType() != CellType.NUMERIC || secondCell.getNumericCellValue() < 0) {
errors.add(String.format("Row %d: Second cell is empty, not a number, or negative", i + 1));
// Skip the rest of the validation
continue;
}
}
// If there are errors, write them to a new sheet
if (errors.size() > 0) {
XSSFSheet errorSheet = workbook.createSheet("Errors");
int rowIdx = 0;
for (String error : errors) {
XSSFRow row = errorSheet.createRow(rowIdx++);
XSSFCell errorCell = row.createCell(0);
errorCell.setCellValue(error);
}
}
// Write the results to a new file
FileOutputStream outputStream = new FileOutputStream(outputFile);
workbook.write(outputStream);
outputStream.close();
System.out.println("Done");
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
此代码假定您的Excel文件有一个名为Sheet1的工作表,其中第一列是字符串和第二列是数字。导入Excel文件后,它会对该工作表进行验证,并将错误信息写入新的工作表中。最后,它将结果写回新文件。
请注意,此示例仅提供了一种简单实现方法。实际应用程序需要根据您的特定需求进行调整。这里只是为了帮助您开始,还需要自己对代码进行深度优化和优化。
相关推荐
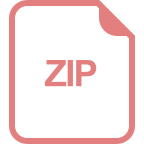












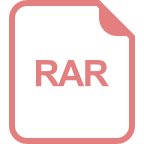