java excel转图片
时间: 2023-08-04 21:14:24 浏览: 37
要将 Excel 中的表格转换为图片,可以使用 Apache POI 和 Java 的图形库。以下是一个示例代码:
```java
import org.apache.poi.hssf.usermodel.*;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.WorkbookFactory;
import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.File;
import javax.imageio.ImageIO;
public class ExcelToImage {
public static void main(String[] args) {
try {
// 读取 Excel 文件
Workbook workbook = WorkbookFactory.create(new File("input.xls"));
HSSFSheet sheet = (HSSFSheet) workbook.getSheetAt(0);
// 计算表格的宽度和高度
int width = 0;
int height = 0;
for (int i = sheet.getFirstRowNum(); i <= sheet.getLastRowNum(); i++) {
HSSFRow row = sheet.getRow(i);
if (row != null) {
int rowWidth = 0;
int rowHeight = 0;
for (int j = row.getFirstCellNum(); j <= row.getLastCellNum(); j++) {
HSSFCell cell = row.getCell(j);
if (cell != null) {
HSSFRichTextString richTextString = cell.getRichStringCellValue();
if (richTextString != null && richTextString.length() > 0) {
Font font = cell.getCellStyle().getFont(workbook);
int cellWidth = getCellWidth(cell, font);
rowWidth += cellWidth;
rowHeight = rowHeight > cell.getRowHeight() ? rowHeight : cell.getRowHeight();
}
}
}
width = width > rowWidth ? width : rowWidth;
height += rowHeight;
}
}
// 创建 BufferedImage 对象并绘制表格
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
Graphics2D g2d = image.createGraphics();
g2d.setColor(Color.WHITE);
g2d.fillRect(0, 0, width, height);
int y = 0;
for (int i = sheet.getFirstRowNum(); i <= sheet.getLastRowNum(); i++) {
HSSFRow row = sheet.getRow(i);
if (row != null) {
int x = 0;
int rowHeight = 0;
for (int j = row.getFirstCellNum(); j <= row.getLastCellNum(); j++) {
HSSFCell cell = row.getCell(j);
if (cell != null) {
HSSFRichTextString richTextString = cell.getRichStringCellValue();
if (richTextString != null && richTextString.length() > 0) {
Font font = cell.getCellStyle().getFont(workbook);
int cellWidth = getCellWidth(cell, font);
int cellHeight = cell.getRowHeight();
rowHeight = rowHeight > cellHeight ? rowHeight : cellHeight;
drawCell(g2d, richTextString.toString(), font, x, y, cellWidth, cellHeight);
x += cellWidth;
}
}
}
y += rowHeight;
}
}
// 保存为图片
ImageIO.write(image, "png", new File("output.png"));
} catch (Exception e) {
e.printStackTrace();
}
}
// 绘制单元格
private static void drawCell(Graphics2D g2d, String text, Font font, int x, int y, int width, int height) {
g2d.setFont(font);
g2d.setPaint(Color.BLACK);
g2d.drawString(text, x, y + height - 5);
g2d.setPaint(Color.GRAY);
g2d.drawRect(x, y, width, height);
}
// 获取单元格宽度
private static int getCellWidth(HSSFCell cell, Font font) {
int defaultWidth = 64;
int defaultHeight = 20;
int charWidth = font.getSize() * 7 / 10;
int cellWidth = cell.getStringCellValue().getBytes().length * charWidth;
int width = cellWidth > defaultWidth ? cellWidth : defaultWidth;
return width;
}
}
```
此代码将表格转换为 BufferedImage 对象,并绘制在其中。然后将 BufferedImage 对象保存为 PNG 图片。您可以根据需要修改此代码以适应您的情况。
相关推荐
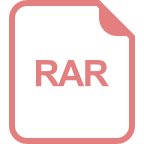
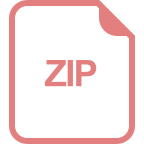





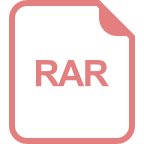
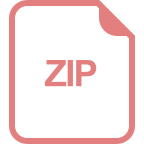
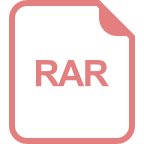