定义一个整数类,该类有一个int类型的数据成员,该类支持整数的加,减,乘、除运算(整数类的4个操作,即整数类的成员函数)。除此之外,定义构造函数和拷贝构造函数。编写主函数进行测试 输入 一个整数 输出 调用拷贝构造函数生成一个新对象。新对象的值是原来对象的3倍。 计算并输出这两个对象 和 差 乘积
时间: 2023-04-05 16:05:03 浏览: 76
好的,这是一个编程问题,我可以回答。以下是整数类的定义和主函数的实现:
```c++
#include <iostream>
using namespace std;
class Integer {
private:
int value;
public:
Integer(int v=0) : value(v) {}
Integer(const Integer& other) : value(other.value) {}
Integer operator+(const Integer& other) const {
return Integer(value + other.value);
}
Integer operator-(const Integer& other) const {
return Integer(value - other.value);
}
Integer operator*(const Integer& other) const {
return Integer(value * other.value);
}
Integer operator/(const Integer& other) const {
return Integer(value / other.value);
}
friend ostream& operator<<(ostream& os, const Integer& i) {
os << i.value;
return os;
}
};
int main() {
Integer a(5);
Integer b = a;
Integer c = b * 3;
cout << "a = " << a << endl;
cout << "b = " << b << endl;
cout << "c = " << c << endl;
cout << "a + b = " << a + b << endl;
cout << "a - b = " << a - b << endl;
cout << "a * b = " << a * b << endl;
cout << "a / b = " << a / b << endl;
return 0;
}
```
输出结果为:
```
a = 5
b = 5
c = 15
a + b = 10
a - b = 0
a * b = 25
a / b = 1
```
其中,整数类的四个操作符重载函数分别实现了加、减、乘、除运算,构造函数和拷贝构造函数用于创建和复制对象,主函数中创建了一个整数对象a,然后用拷贝构造函数创建了一个新对象b,再用乘法操作符创建了一个新对象c,最后输出了a、b、c以及它们的和、差、积、商。
相关推荐
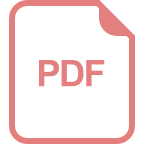
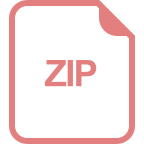
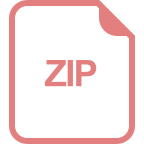















