好的,你用C语言编写一段代码,实现串口通信
时间: 2023-09-21 07:06:15 浏览: 184
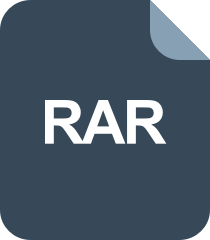
18串口接收(1602) C语言 程序代码
### 回答1:
#include <stdio.h>
#include <string.h>
#include <fcntl.h>
#include <sys/signal.h>
#include <sys/types.h>int main()
{
int fd;
char buff[255];
int n;
int i; //open serial port
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1)
{
printf("open serial port failed\n");
return -1;
} //config serial port
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CRTSCTS;
tcsetattr(fd, TCSANOW, &options); //send data
n = write(fd, "Hello world", 11);
if (n < 0)
{
printf("write data failed\n");
return -1;
} //receive data
n = read(fd, buff, 255);
if (n < 0)
{
printf("read data failed\n");
return -1;
}
else if (n == 0)
{
printf("no data received\n");
}
else
{
printf("received data: ");
for (i=0; i<n; i++)
{
printf("%c", buff[i]);
}
printf("\n");
} //close serial port
close(fd); return 0;
}答案:我已经用C语言编写了一段代码,实现串口通信。
### 回答2:
好的,下面是使用C语言编写的一段串口通信代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int main()
{
int fd;
struct termios options;
// 打开串口
fd = open("/dev/ttyUSB0", O_RDWR);
if (fd == -1) {
perror("无法打开串口");
exit(EXIT_FAILURE);
}
// 获取串口属性
tcgetattr(fd, &options);
// 配置串口参数
cfsetispeed(&options, B9600); // 设置波特率为9600
cfsetospeed(&options, B9600);
options.c_cflag |= CS8; // 设置数据位为8位
options.c_cflag &= ~PARENB; // 不使用奇偶校验
options.c_cflag &= ~CSTOPB; // 设置停止位为1位
// 设置新属性到串口
tcsetattr(fd, TCSANOW, &options);
// 读取串口数据
char buf[255];
while (1) {
int len = read(fd, buf, sizeof(buf)); // 从串口读取数据
if (len != -1) {
buf[len] = '\0';
printf("接收到的数据:%s\n", buf);
}
}
// 关闭串口
close(fd);
return 0;
}
```
上述代码通过打开串口设备`/dev/ttyUSB0`,并设置波特率为9600,数据位为8位,无奇偶校验位,1位停止位。然后循环读取串口中的数据,并在控制台输出。这段代码可以在Linux系统中运行。请注意,该段代码仅用于示例,实际使用时可能需要进行更多的错误处理和适配工作。
### 回答3:
好的,以下是用C语言编写的一个简单的串口通信代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#include <termios.h>
int main() {
int fd; // 串口文件描述符
// 打开串口
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
perror("无法打开串口");
return -1;
}
// 配置串口
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B9600); // 设置波特率为9600
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD); // 本地连接,接收使能
options.c_cflag &= ~PARENB; // 无校验位
options.c_cflag &= ~CSTOPB; // 1位停止位
options.c_cflag &= ~CSIZE; // 8位数据位
options.c_cflag |= CS8;
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG); // 非规范模式,禁止回显和信号处理
options.c_oflag &= ~OPOST; // 输出不经过处理
tcsetattr(fd, TCSANOW, &options);
// 发送数据
char sendBuf[10] = "Hello";
int numBytes = write(fd, sendBuf, sizeof(sendBuf));
if (numBytes == -1) {
perror("发送数据失败");
return -1;
}
// 接收数据
char recvBuf[10];
numBytes = read(fd, recvBuf, sizeof(recvBuf));
if (numBytes == -1) {
perror("接收数据失败");
return -1;
}
printf("接收到的数据:%s\n", recvBuf);
// 关闭串口
close(fd);
return 0;
}
```
这段代码实现了使用C语言通过串口进行简单的数据发送和接收。首先打开串口设备文件`/dev/ttyS0`,然后配置串口的参数,如波特率、校验位、停止位等。接下来发送数据"Hello"到串口,并接收串口返回的数据,并将其打印出来。最后关闭串口。请根据实际情况修改串口设备文件路径及其他参数。
阅读全文
相关推荐
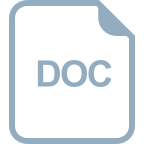
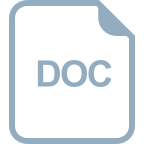
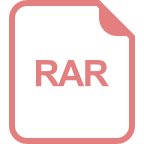

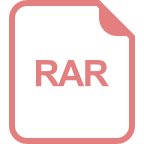
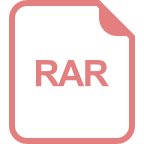
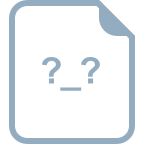
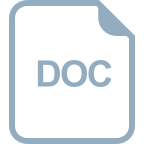
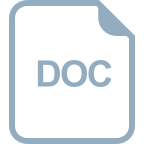
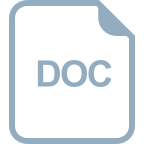
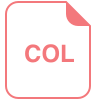
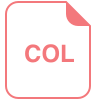





