import javax.swing.*; import java.awt.*; public class DrawCircle extends JPanel implements Runnable{ int angle=0; Thread t; public DrawCircle(){ this.setPreferredSize(new Dimension(500,500)); this.setBackground(Color.blue); t=new Thread(this); t.start(); } public void paintComponent(Graphics g){ super.paintComponent(g); g.fillArc(50, 50, 300, 300, 0, angle); } public static void main(String args[]){ JFrame f=new JFrame("画扇形"); f.getContentPane().add(new DrawCircle()); f.pack(); f.setVisible(true); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } @Override public void run() { // TODO Auto-generated method stub try{ for(int i=0;i<10;i++){ Thread.sleep(100); angle=angle+30; repaint();//重画 重新调用paintComponent } }catch(Exception ex){ } } }
时间: 2024-01-18 14:03:02 浏览: 64
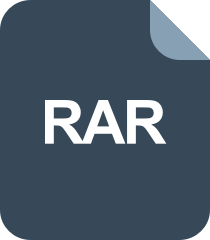
JAVA 程序 源代码 import java.applet.*;import java.awt.*
这是一个Java程序,用于在窗口中绘制一个圆形扇形。程序中使用了JPanel和Thread两个类来实现动态绘制效果。
程序的主要代码如下:
```
import javax.swing.*;
import java.awt.*;
public class DrawCircle extends JPanel implements Runnable {
int angle = 0;
Thread t;
public DrawCircle() {
this.setPreferredSize(new Dimension(500,500));
this.setBackground(Color.blue);
t = new Thread(this);
t.start();
}
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.fillArc(50, 50, 300, 300, 0, angle);
}
public static void main(String args[]) {
JFrame f = new JFrame("画扇形");
f.getContentPane().add(new DrawCircle());
f.pack();
f.setVisible(true);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
@Override
public void run() {
try {
for(int i = 0; i < 10; i++) {
Thread.sleep(100);
angle = angle + 30;
repaint(); //重画 重新调用paintComponent
}
} catch(Exception ex) {
}
}
}
```
程序中首先创建了一个JFrame对象,用于显示绘制结果。然后创建一个DrawCircle对象作为JPanel容器,设置其大小和背景色,并将其添加到JFrame中。接着创建一个新的线程对象,并通过start方法启动该线程。线程的主体是run方法,在其中使用了for循环来控制圆形扇形的绘制,每次循环都休眠100ms,并更新扇形的角度。最后调用JPanel的repaint方法,重新绘制整个扇形。程序中使用了try-catch结构来捕获异常,保证程序的稳定性。
这个程序的运行结果是在一个蓝色的窗口中绘制出一个不断变化的圆形扇形。
阅读全文
相关推荐
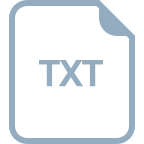
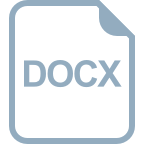

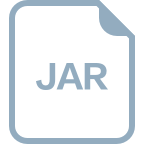
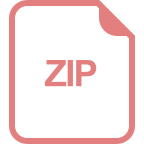
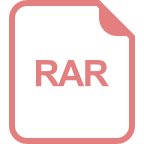
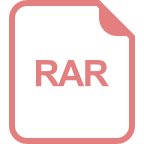
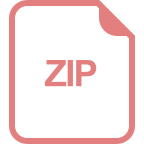
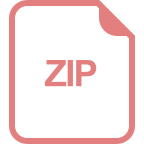
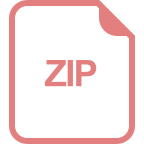
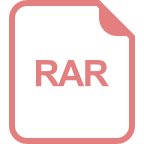
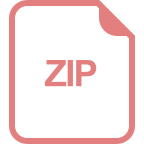
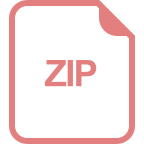
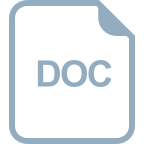
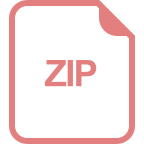