生命游戏使用C++类和对象以及easyx图形库实现
时间: 2023-12-14 19:02:28 浏览: 33
生命游戏是一种基于细胞自动机理论的游戏,它的核心是对生命细胞的状态进行模拟和演化。为了实现这个游戏,我们可以使用C++的类和对象以及easyx图形库来进行开发。
首先,我们可以使用一个Cell类来表示每一个细胞,其中包含细胞的位置、状态等属性以及对应的操作方法,比如更新状态、绘制等。我们还可以设计一个Game类来管理整个游戏的逻辑,包括创建和初始化细胞、更新细胞状态、绘制游戏界面等。
在使用easyx进行图形化界面开发时,我们可以利用easyx提供的绘图函数和事件处理函数,比如用circle函数绘制圆形表示细胞,用mousemsg函数响应鼠标点击事件等。
下面是一个简单的示例代码,展示了如何使用C++类和对象以及easyx库来实现生命游戏:
```cpp
#include <graphics.h>
#include <conio.h>
const int CELL_SIZE = 20; // 细胞大小
const int ROWS = 20; // 行数
const int COLS = 20; // 列数
class Cell {
public:
Cell(int x, int y);
void update(Cell cells[][COLS]);
void draw();
bool isAlive() { return m_alive; }
void setAlive(bool alive) { m_alive = alive; }
private:
int m_x;
int m_y;
bool m_alive;
};
class Game {
public:
Game();
void run();
private:
Cell m_cells[ROWS][COLS];
void init();
void update();
void draw();
void handleMouse();
};
Cell::Cell(int x, int y) {
m_x = x;
m_y = y;
m_alive = false;
}
void Cell::update(Cell cells[][COLS]) {
int count = 0;
for (int i = m_x - 1; i <= m_x + 1; i++) {
for (int j = m_y - 1; j <= m_y + 1; j++) {
if (i >= 0 && i < ROWS && j >= 0 && j < COLS && cells[i][j].m_alive) {
count++;
}
}
}
if (m_alive) {
count--;
if (count < 2 || count > 3) {
m_alive = false;
}
} else {
if (count == 3) {
m_alive = true;
}
}
}
void Cell::draw() {
if (m_alive) {
setfillcolor(YELLOW);
} else {
setfillcolor(BLACK);
}
setlinecolor(WHITE);
fillcircle(m_x * CELL_SIZE + CELL_SIZE / 2, m_y * CELL_SIZE + CELL_SIZE / 2, CELL_SIZE / 2);
}
Game::Game() {
init();
}
void Game::run() {
while (true) {
update();
draw();
handleMouse();
delay(100);
}
}
void Game::init() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
m_cells[i][j] = Cell(i, j);
}
}
}
void Game::update() {
Cell tmp[ROWS][COLS];
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
tmp[i][j] = m_cells[i][j];
tmp[i][j].update(m_cells);
}
}
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
m_cells[i][j] = tmp[i][j];
}
}
}
void Game::draw() {
cleardevice();
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
m_cells[i][j].draw();
}
}
}
void Game::handleMouse() {
MOUSEMSG mouseMsg = GetMouseMsg();
if (mouseMsg.uMsg == WM_LBUTTONDOWN) {
int x = mouseMsg.x / CELL_SIZE;
int y = mouseMsg.y / CELL_SIZE;
m_cells[x][y].setAlive(true);
} else if (mouseMsg.uMsg == WM_RBUTTONDOWN) {
int x = mouseMsg.x / CELL_SIZE;
int y = mouseMsg.y / CELL_SIZE;
m_cells[x][y].setAlive(false);
}
}
int main() {
initgraph(CELL_SIZE * COLS, CELL_SIZE * ROWS);
Game game;
game.run();
getch();
closegraph();
return 0;
}
```
这段代码实现了一个简单的生命游戏,在游戏开始时,我们初始化了一个20行20列的细胞矩阵。在游戏运行过程中,我们不断更新细胞的状态,并将其绘制出来,从而实现了生命游戏的模拟。另外,我们还添加了鼠标点击事件的处理,当用户点击鼠标左键时,会将对应位置的细胞设置为存活状态;当用户点击鼠标右键时,会将对应位置的细胞设置为死亡状态。
相关推荐
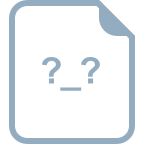













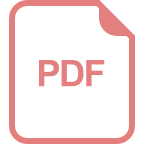
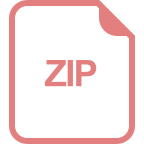