用 python 代码编写一个人脸表情识别的程序
时间: 2023-05-20 12:02:42 浏览: 152
好的,以下是一个简单的 Python 人脸表情识别程序:
```python
import cv2
import numpy as np
from keras.models import load_model
# 加载训练好的模型
model = load_model('model.h5')
# 加载人脸分类器
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
# 定义表情标签
emotions = ['Angry', 'Disgust', 'Fear', 'Happy', 'Neutral', 'Sad', 'Surprise']
# 打开摄像头
cap = cv2.VideoCapture(0)
while True:
# 读取摄像头中的图像
ret, frame = cap.read()
# 转换为灰度图像
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 检测人脸
faces = face_cascade.detectMultiScale(gray, 1.3, 5)
# 对每个检测到的人脸进行表情识别
for (x, y, w, h) in faces:
# 提取人脸图像
face = gray[y:y+h, x:x+w]
# 调整图像大小
face = cv2.resize(face, (48, 48))
# 归一化像素值
face = face / 255.0
# 转换为四维张量
face = np.expand_dims(face, axis=0)
face = np.expand_dims(face, axis=-1)
# 进行表情识别
prediction = model.predict(face)[0]
# 获取最大概率的表情标签
label = emotions[np.argmax(prediction)]
# 在人脸周围绘制矩形框和表情标签
cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 255, 0), 2)
cv2.putText(frame, label, (x, y-10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, (0, 255, 0), 2)
# 显示图像
cv2.imshow('Face Expression Recognition', frame)
# 按下 q 键退出程序
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放摄像头并关闭窗口
cap.release()
cv2.destroyAllWindows()
```
请注意,这只是一个简单的示例程序,实际上,人脸表情识别是一个非常复杂的问题,需要大量的数据和计算资源来训练一个准确的模型。
阅读全文
相关推荐
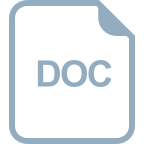
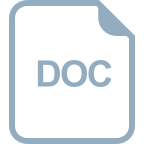
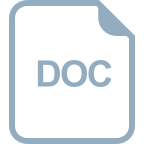






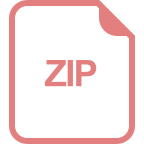
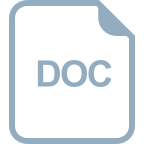
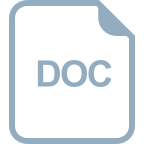
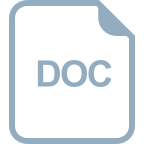
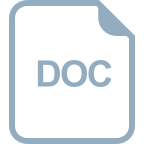
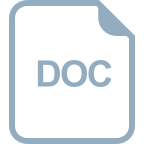
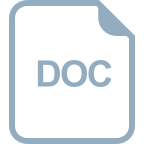

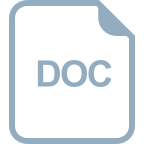
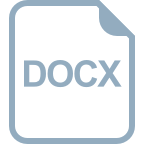