Python写mr
时间: 2023-08-27 08:10:40 浏览: 68
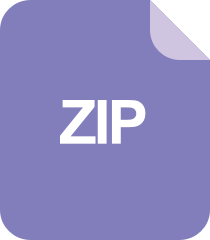
python编写MR
MapReduce是一种分布式计算模型,Python可以通过Hadoop Streaming实现MapReduce功能。以下是一个使用Python实现的简单MapReduce示例:
假设我们有一个包含数字的文本文件,我们想要计算每个数字出现的次数。
首先,我们需要编写一个map.py脚本来实现map函数的功能。map.py的代码如下所示:
```
#!/usr/bin/env python
import sys
for line in sys.stdin:
line = line.strip()
words = line.split()
for word in words:
print('%s\t%s' % (word, 1))
```
这个脚本将输入的每一行文本拆分成单个数字,并将每个数字输出为键值对(数字,1)。
接下来,我们需要编写一个reduce.py脚本来实现reduce函数的功能。reduce.py的代码如下所示:
```
#!/usr/bin/env python
import sys
current_word = None
current_count = 0
word = None
for line in sys.stdin:
line = line.strip()
word, count = line.split('\t', 1)
try:
count = int(count)
except ValueError:
continue
if current_word == word:
current_count += count
else:
if current_word:
print('%s\t%s' % (current_word, current_count))
current_count = count
current_word = word
if current_word == word:
print('%s\t%s' % (current_word, current_count))
```
这个脚本将接收到的键值对按键进行排序,并且累加相同键的值。最后输出每个数字出现的次数。
最后,我们可以通过Hadoop Streaming来运行MapReduce作业。假设我们的输入文件名为input.txt,输出文件名为output.txt,我们可以使用以下命令来运行:
```
hadoop jar /path/to/hadoop-streaming.jar \
-file /path/to/map.py -mapper /path/to/map.py \
-file /path/to/reduce.py -reducer /path/to/reduce.py \
-input /path/to/input.txt -output /path/to/output.txt
```
这个命令将使用MapReduce对输入文件进行处理,并将结果输出到指定的输出文件中。
阅读全文
相关推荐
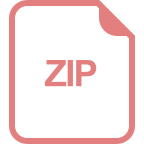
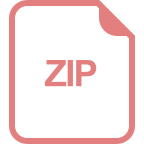















