编写一个C++程序,要求2-3 商店销售某一商品,商店每天公布统一的折扣(discount)。同时允许销售人员在销售时灵活掌握售价(price),在此基础上,对一次购10件以上者,还可以享受9.8折优惠。现已知当天3名销货员的销售情况为: 销货员号(num) 销货件数(quantity) 销货单价(price) 101 5 23.5 102 12 24.56 103 100 21.5 编程计算出当日此商品的总销售款sum,以及每件商品的平均售价。要求用静态数据成员和静态成员函数。
时间: 2024-02-10 18:04:48 浏览: 154
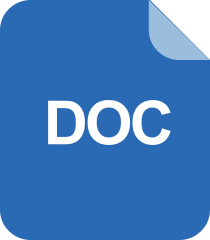
Dev-C++ 编译多文件程序的方法&安装使用教程

以下是一个简单的 C++ 程序,实现了上述要求:
```cpp
#include <iostream>
using namespace std;
class Salesman {
public:
Salesman(int num, int quantity, double price) {
this->num = num;
this->quantity = quantity;
this->price = price;
sum += quantity * price * discount();
total_quantity += quantity;
total_price += price * quantity;
count++;
}
static double discount() {
return count >= 10 ? 0.98 : 1.0;
}
static double avg_price() {
return total_price / total_quantity;
}
static double total_sales() {
return sum;
}
private:
int num;
int quantity;
double price;
static double sum;
static int count;
static int total_quantity;
static double total_price;
};
double Salesman::sum = 0;
int Salesman::count = 0;
int Salesman::total_quantity = 0;
double Salesman::total_price = 0;
int main() {
Salesman s1(101, 5, 23.5);
Salesman s2(102, 12, 24.56);
Salesman s3(103, 100, 21.5);
cout << "Total sales: " << Salesman::total_sales() << endl;
cout << "Average price: " << Salesman::avg_price() << endl;
return 0;
}
```
程序定义了一个 `Salesman` 类,包含了销售员号、销售件数、销售单价三个数据成员和一个构造函数、一个静态成员函数 `discount()` 和三个静态成员函数 `avg_price()`、`total_sales()` 和 `discount()`。在构造函数中,根据销售件数和单价计算销售额并存入 `sum` 中,并将销售件数、销售额等数据存入对应的静态数据成员中。在 `discount()` 函数中,根据销售员数是否达到 10 人判断是否享受 9.8 折优惠。在 `avg_price()` 函数中,计算并返回每件商品的平均售价。在 `total_sales()` 函数中,返回当日此商品的总销售款。
在 `main()` 函数中,定义了三个销售员对象,分别记录销售情况。最后输出总销售款和平均售价,通过调用 `Salesman::total_sales()` 和 `Salesman::avg_price()` 静态函数实现。
阅读全文
相关推荐
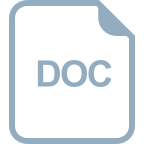
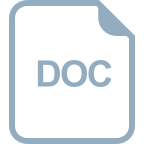
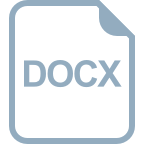
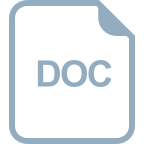
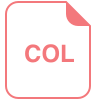










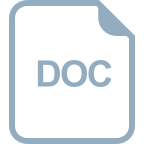
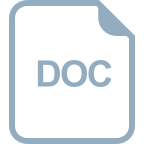