c ++小型企业工资管理系统中的查询系统能根据工号或姓名查询工资信息代码
时间: 2024-05-04 14:22:17 浏览: 102
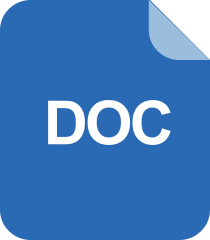
小型公司工资管理系统C++源代码.doc

以下是一个简单的C++程序,可以实现根据工号或姓名查询工资信息的功能。
```c++
#include <iostream>
#include <string>
using namespace std;
struct Employee {
int id; // 工号
string name; // 姓名
float salary; // 工资
};
// 初始化员工信息
void initEmployees(Employee employees[], int n) {
for (int i = 0; i < n; i++) {
employees[i].id = i + 1;
employees[i].name = "员工" + to_string(i + 1);
employees[i].salary = 5000 + i * 500;
}
}
// 根据工号或姓名查询员工信息
void queryEmployee(Employee employees[], int n) {
int choice;
cout << "请选择查询方式:1.按工号查询 2.按姓名查询" << endl;
cin >> choice;
if (choice == 1) {
int id;
cout << "请输入工号:";
cin >> id;
for (int i = 0; i < n; i++) {
if (employees[i].id == id) {
cout << "工号:" << employees[i].id << endl;
cout << "姓名:" << employees[i].name << endl;
cout << "工资:" << employees[i].salary << endl;
return;
}
}
cout << "未找到工号为" << id << "的员工信息" << endl;
} else if (choice == 2) {
string name;
cout << "请输入姓名:";
cin >> name;
for (int i = 0; i < n; i++) {
if (employees[i].name == name) {
cout << "工号:" << employees[i].id << endl;
cout << "姓名:" << employees[i].name << endl;
cout << "工资:" << employees[i].salary << endl;
return;
}
}
cout << "未找到姓名为" << name << "的员工信息" << endl;
} else {
cout << "无效的选项" << endl;
}
}
int main() {
const int n = 5;
Employee employees[n];
initEmployees(employees, n);
queryEmployee(employees, n);
return 0;
}
```
程序中定义了一个结构体 `Employee` 表示员工信息,包含工号、姓名和工资三个字段。`initEmployees` 函数用于初始化员工信息,这里简单地按照工号、姓名和工资的规律生成了员工信息。`queryEmployee` 函数根据用户选择的查询方式(按工号或按姓名)输入查询条件,并遍历员工信息数组查找匹配的员工信息。如果找到了,则输出员工信息;否则输出未找到信息。在 `main` 函数中调用了 `initEmployees` 和 `queryEmployee` 函数。
阅读全文
相关推荐
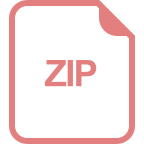
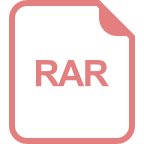
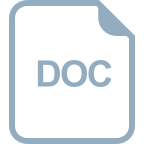
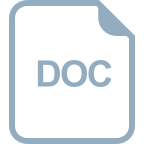
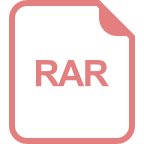
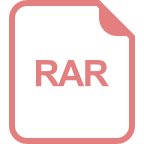
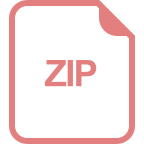
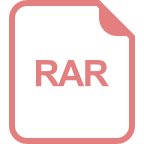
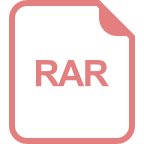
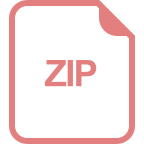
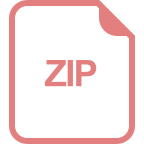
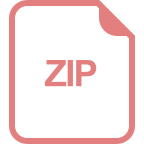
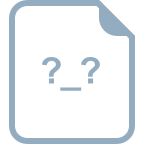
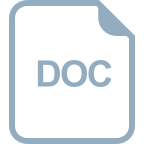
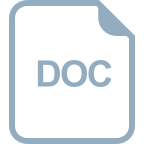
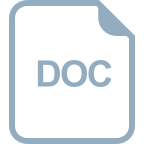