写函数,计算传入字符串中的【数字】、【字母】、【空格】和【其他】的个数
时间: 2023-08-31 17:39:18 浏览: 61
### 回答1:
好的,我可以为您编写一个函数来计算传入字符串中的数字、字母、空格和其他字符的个数。
以下是函数的代码:
```python
def count_chars(string):
letters = 0
digits = 0
spaces = 0
others = 0
for char in string:
if char.isalpha():
letters += 1
elif char.isdigit():
digits += 1
elif char.isspace():
spaces += 1
else:
others += 1
print("数字个数:", digits)
print("字母个数:", letters)
print("空格个数:", spaces)
print("其他字符个数:", others)
```
使用该函数,您可以计算传入任何字符串中数字、字母、空格和其他字符的个数。例如,以下是使用该函数计算字符串 "Hello World 123!" 中字符的个数的示例:
```python
count_chars("Hello World 123!")
```
输出会是:
```
数字个数: 3
字母个数: 10
空格个数: 2
其他字符个数: 1
```
### 回答2:
要写出这个函数,首先需要对传入的字符串进行遍历,然后通过判断字符的ASCII码来确定其所属的类型。
可以定义四个变量:num_count(数字计数)、alpha_count(字母计数)、space_count(空格计数)、other_count(其他字符计数),并初始化为0。
接下来使用循环遍历传入的字符串中的每一个字符,对每个字符进行判断:
1. 如果字符是数字,即ASCII码在48到57之间,那么num_count加1。
2. 如果字符是字母,即ASCII码在65到90和97到122之间,那么alpha_count加1。
3. 如果字符是空格,即ASCII码为32,那么space_count加1。
4. 如果字符不属于以上三种情况,那么other_count加1。
最后,将num_count、alpha_count、space_count和other_count的值返回即可得到各类型字符在字符串中的个数。
下面是代码示例:
```python
def count_chars(string):
num_count = 0
alpha_count = 0
space_count = 0
other_count = 0
for char in string:
if 48 <= ord(char) <= 57:
num_count += 1
elif (65 <= ord(char) <= 90) or (97 <= ord(char) <= 122):
alpha_count += 1
elif ord(char) == 32:
space_count += 1
else:
other_count += 1
return num_count, alpha_count, space_count, other_count
```
示例调用代码:
```python
string = "Hello World! 123"
num_count, alpha_count, space_count, other_count = count_chars(string)
print("数字个数:", num_count)
print("字母个数:", alpha_count)
print("空格个数:", space_count)
print("其他字符个数:", other_count)
```
输出结果:
```
数字个数: 3
字母个数: 10
空格个数: 2
其他字符个数: 1
```
### 回答3:
可以编写一个函数,计算传入字符串中的数字、字母、空格和其他字符的个数。
以下是一个示例代码:
```python
def count_chars(string):
digit_count = 0
letter_count = 0
space_count = 0
other_count = 0
for char in string:
if char.isdigit():
digit_count += 1
elif char.isalpha():
letter_count += 1
elif char.isspace():
space_count += 1
else:
other_count += 1
return digit_count, letter_count, space_count, other_count
string = input('请输入一个字符串:')
digit_count, letter_count, space_count, other_count = count_chars(string)
print('数字个数为:', digit_count)
print('字母个数为:', letter_count)
print('空格个数为:', space_count)
print('其他字符个数为:', other_count)
```
可以通过输入一个字符串,然后调用count_chars函数得到数字、字母、空格和其他字符的个数,并打印出来。
相关推荐
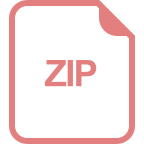
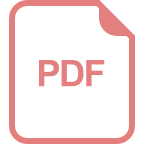














