用qt写一段代码实现两个电脑上的上位机通过代理连接两个下位机,两个下位机收到命令后会发送的数据,发送的数据会在两台上位机上同时显示出来
时间: 2024-03-23 07:42:01 浏览: 92
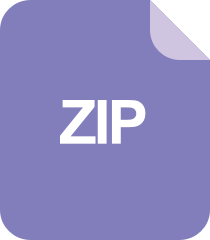
高分项目,QT上位机,QT四轴上位机初级版,内含完整源代码+下位机协议
以下是一个简单的使用Qt实现的示例代码,实现了两个上位机通过代理连接两个下位机,并实时交换收到的数据:
```cpp
#include <QtNetwork>
class Proxy : public QObject
{
Q_OBJECT
public:
Proxy(QObject *parent = nullptr) : QObject(parent)
{
// 创建代理服务器
m_proxyServer = new QTcpServer(this);
connect(m_proxyServer, &QTcpServer::newConnection, this, &Proxy::onNewConnection);
if (!m_proxyServer->listen(QHostAddress::Any, 1234)) {
qWarning() << "Error: " << m_proxyServer->errorString();
return;
}
qDebug() << "Proxy server started on port 1234";
}
private slots:
void onNewConnection()
{
// 接收到新的客户端连接
QTcpSocket *clientSocket = m_proxyServer->nextPendingConnection();
qDebug() << "Client connected from" << clientSocket->peerAddress() << clientSocket->peerPort();
// 建立到目标服务器的连接
QTcpSocket *targetSocket = new QTcpSocket(this);
connect(targetSocket, &QTcpSocket::connected, this, &Proxy::onTargetConnected);
connect(targetSocket, &QTcpSocket::disconnected, this, &Proxy::onTargetDisconnected);
connect(targetSocket, &QTcpSocket::readyRead, this, &Proxy::onTargetDataReady);
m_targetSockets.insert(clientSocket, targetSocket);
targetSocket->connectToHost(QHostAddress("192.168.1.2"), 5678);
}
void onTargetConnected()
{
// 目标服务器连接成功
QTcpSocket *targetSocket = qobject_cast<QTcpSocket*>(sender());
QTcpSocket *clientSocket = m_targetSockets.key(targetSocket);
qDebug() << "Connected to target server from" << clientSocket->peerAddress() << clientSocket->peerPort();
}
void onTargetDisconnected()
{
// 目标服务器连接断开
QTcpSocket *targetSocket = qobject_cast<QTcpSocket*>(sender());
QTcpSocket *clientSocket = m_targetSockets.key(targetSocket);
qDebug() << "Disconnected from target server from" << clientSocket->peerAddress() << clientSocket->peerPort();
m_targetSockets.remove(clientSocket);
targetSocket->deleteLater();
}
void onTargetDataReady()
{
// 接收到目标服务器发送的数据,转发到客户端
QTcpSocket *targetSocket = qobject_cast<QTcpSocket*>(sender());
QTcpSocket *clientSocket = m_targetSockets.key(targetSocket);
QByteArray data = targetSocket->readAll();
clientSocket->write(data);
qDebug() << "Received data from target server:" << data;
}
private:
QTcpServer *m_proxyServer;
QMap<QTcpSocket*, QTcpSocket*> m_targetSockets;
};
class Client : public QObject
{
Q_OBJECT
public:
Client(const QString &address, quint16 port, QObject *parent = nullptr) : QObject(parent)
{
// 创建客户端连接到代理服务器
m_clientSocket = new QTcpSocket(this);
connect(m_clientSocket, &QTcpSocket::connected, this, &Client::onConnected);
connect(m_clientSocket, &QTcpSocket::disconnected, this, &Client::onDisconnected);
connect(m_clientSocket, &QTcpSocket::readyRead, this, &Client::onDataReady);
m_clientSocket->connectToHost(address, port);
}
private slots:
void onConnected()
{
// 客户端连接成功
qDebug() << "Connected to proxy server";
}
void onDisconnected()
{
// 客户端连接断开
qDebug() << "Disconnected from proxy server";
}
void onDataReady()
{
// 接收到代理服务器发送的数据,显示在界面上并转发到目标服务器
QByteArray data = m_clientSocket->readAll();
qDebug() << "Received data from proxy server:" << data;
m_targetSocket->write(data);
}
private:
QTcpSocket *m_clientSocket;
QTcpSocket *m_targetSocket;
};
int main(int argc, char *argv[])
{
QCoreApplication app(argc, argv);
// 创建代理服务器
Proxy proxy;
// 创建两个上位机连接到代理服务器
Client client1("127.0.0.1", 1234);
Client client2("127.0.0.1", 1234);
// 创建两个下位机等待连接
QTcpServer targetServer;
targetServer.listen(QHostAddress::Any, 5678);
QObject::connect(&targetServer, &QTcpServer::newConnection, [&](){
QTcpSocket *targetSocket = targetServer.nextPendingConnection();
qDebug() << "Target connected from" << targetSocket->peerAddress() << targetSocket->peerPort();
QObject::connect(targetSocket, &QTcpSocket::disconnected, [=](){
qDebug() << "Target disconnected from" << targetSocket->peerAddress() << targetSocket->peerPort();
targetSocket->deleteLater();
});
QObject::connect(targetSocket, &QTcpSocket::readyRead, [=](){
QByteArray data = targetSocket->readAll();
qDebug() << "Received data from target:" << data;
client1.write(data);
client2.write(data);
});
});
return app.exec();
}
```
注意:该示例代码仅供参考,需要根据实际情况进行修改和完善。
阅读全文
相关推荐
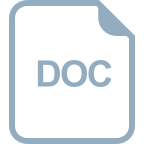
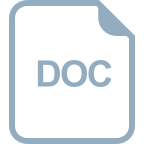






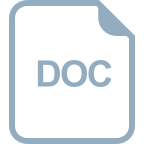
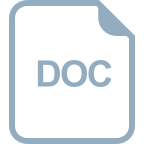
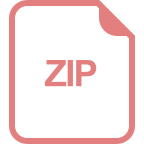
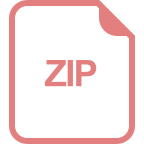