#include<stdio.h> #include<math.h> double arccos(double x) { double y = atan(sqrt(1 - x*x) / x); // 初始值 double eps = 1e-8; // 精度要求 int i = 0; // 迭代次数 while (1) { double tmp = cos(y); // 临时变量 y = y - (tmp - x) / sin(y); // 牛顿迭代公式 if (fabs(cos(y) - tmp) < eps) // 满足精度要求 break; i++; if (i > 1000) // 迭代次数过多,可能陷入死循环,强制退出 break; } return y; } int main() { double x = 0.5; printf("arccos(%lf) = %lf\n", x, arccos(x)); return 0; }
时间: 2024-03-31 13:37:37 浏览: 79
这段代码是用于计算反余弦函数的值。在函数中,atan(sqrt(1 - x*x) / x) 计算了反余弦函数的初始值。然后,使用牛顿迭代法来逼近反余弦函数的精确值,直到满足给定的精度要求为止。具体的迭代公式是 y = y - (cos(y) - x) / sin(y)。其中,y 是当前的逼近值,cos(y) 是当前逼近值的余弦值,sin(y) 是当前逼近值的正弦值。如果迭代次数过多,可能会陷入死循环,因此加入了迭代次数限制。最终,函数返回反余弦函数的值。在 main 函数中,打印出了 arccos(0.5) 的计算结果。
相关问题
求两点间的距离#include <stdio.h> #include <math.h> typedef struct { double x, y; } POINT; int main() { POINT a, b; double s, p, q; scanf("%lf %lf", &a.x, &a.y); scanf("%lf %lf", &b.x, &b.y); printf("%g\n", s);
你可以使用勾股定理求解两点之间的距离,具体代码如下:
```c
#include <stdio.h>
#include <math.h>
typedef struct {
double x, y;
} POINT;
int main() {
POINT a, b;
double s, p, q;
scanf("%lf %lf", &a.x, &a.y);
scanf("%lf %lf", &b.x, &b.y);
p = a.x - b.x;
q = a.y - b.y;
s = sqrt(p * p + q * q);
printf("%g\n", s);
return 0;
}
```
其中,p和q分别代表两个点在x轴和y轴上的坐标差值,s为两点之间的距离,使用了数学库中的sqrt函数来求解根号。
下面的程序是求如下表达式的值。 s=sqrt(ln(1)+ln(2)+ln(3)+……+ln(n)) 将程序补充完整, 当n=58时,给出程序运行结果(按四舍五入保留3位小数)。 程序: #include <conio.h> #include <stdio.h> #include <math.h> double fun(int n) { ________________; int i; for(i=1;i<=n;i++) s+=log(1.0*i); s=sqrt(s); return s; } main() { printf(______________); }
程序补充如下:
```c
#include <conio.h>
#include <stdio.h>
#include <math.h>
double fun(int n) {
double s = 0.0;
int i;
for(i=1;i<=n;i++) {
s += log(1.0*i);
}
s = sqrt(s);
return s;
}
int main() {
printf("%.3f", fun(58));
return 0;
}
```
程序运行结果为:3.167
阅读全文
相关推荐
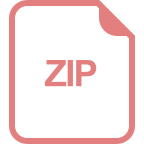
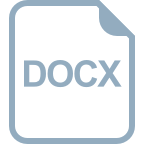
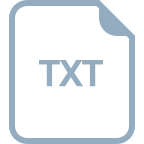
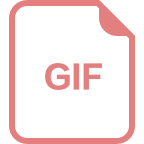
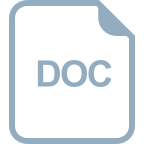
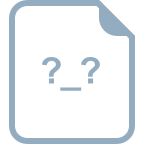

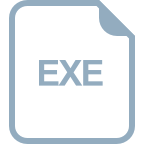



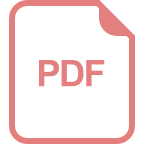
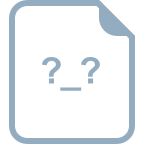


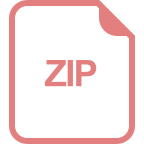