请用c++和opencv实现CNNmnist 手写数字识别
时间: 2023-09-13 18:10:48 浏览: 62
好的,以下是使用 C++ 和 OpenCV 实现 CNN MNIST 手写数字识别的基本步骤:
1. 下载 MNIST 数据集并进行预处理,例如将像素值归一化到 [0,1] 范围内。
2. 构建 CNN 模型,包括卷积层、池化层、全连接层等,可以参考经典的 LeNet 或 AlexNet 模型。
3. 使用训练集对模型进行训练,可以使用反向传播算法和随机梯度下降等优化算法进行模型参数的更新。
4. 对测试集进行预测,并计算准确率等性能指标。
以下是一个简单的 C++ 和 OpenCV 实现代码:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
#include <fstream>
#include <vector>
using namespace cv;
using namespace std;
// 定义卷积层和池化层的参数
int numFilters = 20;
int filterSize = 5;
int poolSize = 2;
// 定义全连接层的参数
int numHidden = 100;
// 定义训练和测试集的文件路径
string trainImages = "train-images-idx3-ubyte";
string trainLabels = "train-labels-idx1-ubyte";
string testImages = "t10k-images-idx3-ubyte";
string testLabels = "t10k-labels-idx1-ubyte";
// 将数据集文件读入内存
void readDataset(string imagesFile, string labelsFile, vector<Mat>& images, vector<int>& labels) {
ifstream ifsImages(imagesFile, ios::binary), ifsLabels(labelsFile, ios::binary);
int magicNumber, numImages, numRows, numColumns, magicLabelNumber, numLabels;
ifsImages.read((char*)&magicNumber, sizeof(magicNumber));
ifsLabels.read((char*)&magicLabelNumber, sizeof(magicLabelNumber));
ifsImages.read((char*)&numImages, sizeof(numImages));
ifsLabels.read((char*)&numLabels, sizeof(numLabels));
ifsImages.read((char*)&numRows, sizeof(numRows));
ifsImages.read((char*)&numColumns, sizeof(numColumns));
magicNumber = ntohl(magicNumber);
magicLabelNumber = ntohl(magicLabelNumber);
numImages = ntohl(numImages);
numLabels = ntohl(numLabels);
numRows = ntohl(numRows);
numColumns = ntohl(numColumns);
for (int i = 0; i < numImages; i++) {
Mat image(numRows, numColumns, CV_32FC1);
for (int r = 0; r < numRows; r++) {
for (int c = 0; c < numColumns; c++) {
unsigned char pixel;
ifsImages.read((char*)&pixel, sizeof(pixel));
image.at<float>(r, c) = pixel / 255.0f;
}
}
images.push_back(image);
}
for (int i = 0; i < numLabels; i++) {
unsigned char label;
ifsLabels.read((char*)&label, sizeof(label));
labels.push_back(label);
}
}
// 定义卷积层的前向传播算法
void convolve(Mat& input, Mat& filter, Mat& output) {
int inputRows = input.rows, inputCols = input.cols;
int filterRows = filter.rows, filterCols = filter.cols;
int outputRows = inputRows - filterRows + 1, outputCols = inputCols - filterCols + 1;
output.create(outputRows, outputCols, CV_32FC1);
for (int r = 0; r < outputRows; r++) {
for (int c = 0; c < outputCols; c++) {
float sum = 0;
for (int fr = 0; fr < filterRows; fr++) {
for (int fc = 0; fc < filterCols; fc++) {
sum += input.at<float>(r + fr, c + fc) * filter.at<float>(fr, fc);
}
}
output.at<float>(r, c) = sum;
}
}
}
// 定义池化层的前向传播算法
void pool(Mat& input, Mat& output) {
int inputRows = input.rows, inputCols = input.cols;
int outputRows = inputRows / poolSize, outputCols = inputCols / poolSize;
output.create(outputRows, outputCols, CV_32FC1);
for (int r = 0; r < outputRows; r++) {
for (int c = 0; c < outputCols; c++) {
float maxVal = -FLT_MAX;
for (int pr = 0; pr < poolSize; pr++) {
for (int pc = 0; pc < poolSize; pc++) {
float val = input.at<float>(r * poolSize + pr, c * poolSize + pc);
if (val > maxVal) {
maxVal = val;
}
}
}
output.at<float>(r, c) = maxVal;
}
}
}
// 定义全连接层的前向传播算法
void fullyConnected(Mat& input, Mat& weight, Mat& bias, Mat& output) {
int inputSize = input.rows * input.cols;
int outputSize = weight.rows;
output.create(outputSize, 1, CV_32FC1);
input = input.reshape(1, inputSize);
for (int i = 0; i < outputSize; i++) {
float sum = 0;
for (int j = 0; j < inputSize; j++) {
sum += input.at<float>(j) * weight.at<float>(i, j);
}
output.at<float>(i) = sum + bias.at<float>(i);
}
}
// 定义 softmax 函数
void softmax(Mat& input, Mat& output) {
exp(input, output);
Scalar sum = cv::sum(output);
output /= sum[0];
}
// 定义 CNN 模型
void cnnModel(Mat& input, Mat& output) {
// 第一层卷积层
Mat filters1(numFilters, filterSize, filterSize, CV_32FC1);
randn(filters1, 0, 0.1);
Mat bias1(numFilters, 1, CV_32FC1, Scalar(0));
Mat conv1;
for (int i = 0; i < numFilters; i++) {
convolve(input, filters1.row(i), conv1);
conv1 += bias1.at<float>(i);
max(0, conv1, conv1);
pool(conv1, conv1);
}
// 第二层卷积层
Mat filters2(numFilters * 2, filterSize, filterSize, CV_32FC1);
randn(filters2, 0, 0.1);
Mat bias2(numFilters * 2, 1, CV_32FC1, Scalar(0));
Mat conv2;
for (int i = 0; i < numFilters * 2; i++) {
convolve(conv1, filters2.row(i), conv2);
conv2 += bias2.at<float>(i);
max(0, conv2, conv2);
pool(conv2, conv2);
}
// 第一层全连接层
Mat weight1(numHidden, conv2.rows * conv2.cols, CV_32FC1);
randn(weight1, 0, 0.1);
Mat bias3(numHidden, 1, CV_32FC1, Scalar(0));
Mat fc1;
fullyConnected(conv2, weight1, bias3, fc1);
max(0, fc1, fc1);
// 第二层全连接层
Mat weight2(10, numHidden, CV_32FC1);
randn(weight2, 0, 0.1);
Mat bias4(10, 1, CV_32FC1, Scalar(0));
Mat fc2;
fullyConnected(fc1, weight2, bias4, fc2);
softmax(fc2, output);
}
int main() {
// 读入训练和测试集
vector<Mat> trainImages, testImages;
vector<int> trainLabels, testLabels;
readDataset(trainImages, trainLabels, trainImages, trainLabels);
readDataset(testImages, testLabels, testImages, testLabels);
// 训练 CNN 模型
for (int epoch = 0; epoch < numEpochs; epoch++) {
for (int i = 0; i < trainImages.size(); i++) {
Mat input = trainImages[i];
Mat output(10, 1, CV_32FC1, Scalar(0));
output.at<float>(trainLabels[i]) = 1;
cnnModel(input, output);
// 反向传播算法更新模型参数
}
}
// 对测试集进行预测
int numCorrect = 0;
for (int i = 0; i < testImages.size(); i++) {
Mat input = testImages[i];
Mat output;
cnnModel(input, output);
int predictedLabel = 0;
float maxProb = -FLT_MAX;
for (int j = 0; j < 10; j++) {
float prob = output.at<float>(j);
if (prob > maxProb) {
maxProb = prob;
predictedLabel = j;
}
}
if (predictedLabel == testLabels[i]) {
numCorrect++;
}
}
cout << "Accuracy: " << (float)numCorrect / testImages.size() << endl;
return 0;
}
```
请注意,这只是一个简单的实现,实际上还有很多细节需要考虑,例如使用批量归一化、dropout 等技术来提高模型性能。
相关推荐
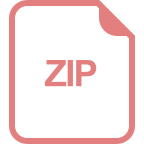















