怎么使用pycuda优化我给出的代码
时间: 2023-12-08 19:07:00 浏览: 125
使用PyCUDA可以利用GPU加速这个求解拉普拉斯方程的过程。以下是使用PyCUDA优化的代码:
```python
import numpy as np
import pycuda.autoinit
import pycuda.driver as drv
from pycuda.compiler import SourceModule
import matplotlib.pyplot as plt
# Set maximum iteration
maxIter = 500
# Set Dimension and delta
lenX = lenY = 20
delta = 1
# Boundary condition
Ttop = 100
Tbottom = 0
Tleft = 0
Tright = 0
# Initial guess of interior grid
Tguess = 30
# Set meshgrid
X, Y = np.meshgrid(np.arange(0, lenX), np.arange(0, lenY))
# Set array size and set the interior value with Tguess
T = np.empty((lenX, lenY), dtype=np.float32)
T.fill(Tguess)
# Set Boundary condition
T[(lenY-1):, :] = Ttop
T[:1, :] = Tbottom
T[:, (lenX-1):] = Tright
T[:, :1] = Tleft
# Define the CUDA kernel for updating the temperature values
mod = SourceModule("""
__global__ void update_T(float *T, int lenX, int lenY)
{
int i = blockDim.x * blockIdx.x + threadIdx.x + 1;
int j = blockDim.y * blockIdx.y + threadIdx.y + 1;
if (i < lenX-1 && j < lenY-1)
{
T[i*lenY+j] = 0.25 * (T[(i+1)*lenY+j] + T[(i-1)*lenY+j] + T[i*lenY+j+1] + T[i*lenY+j-1]);
}
}
""")
# Get the kernel function
update_T = mod.get_function("update_T")
# Set the grid and block sizes
block_size = (16, 16, 1)
grid_size = ((lenX-2)//block_size[0]+1, (lenY-2)//block_size[1]+1, 1)
# Iteration (We assume that the iteration is convergence in maxIter = 500)
print("Please wait for a moment")
for iteration in range(0, maxIter):
update_T(drv.InOut(T), np.int32(lenX), np.int32(lenY), block=block_size, grid=grid_size)
print("Iteration finished")
# Configure the contour
plt.title("Contour of Temperature")
plt.contourf(X, Y, T, 50, cmap=plt.cm.jet)
# Set Colorbar
plt.colorbar()
# Show the result in the plot window
plt.show()
```
这个代码与原始代码非常相似,但是使用了PyCUDA库中的GPU并行计算来加速迭代计算的过程。在这个例子中,我们定义了一个CUDA内核函数来更新温度值,并使用PyCUDA的函数调用语法将数组传递到GPU上进行计算。在每次迭代中,我们使用PyCUDA函数调用来更新温度数组的值。最后,我们使用matplotlib库创建温度的轮廓图。
阅读全文
相关推荐
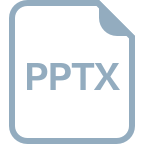
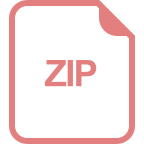
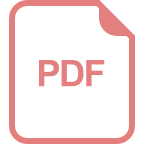
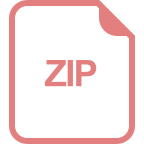
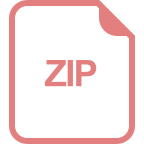
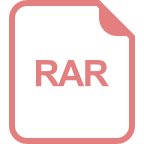
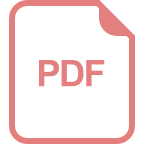
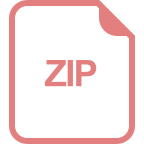
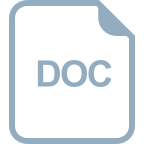
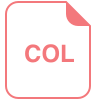
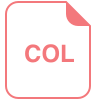
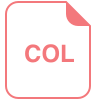
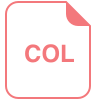
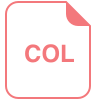



