python写一个word转pdf代码
时间: 2023-02-23 10:51:11 浏览: 59
可以使用 python 中的第三方库 "python-docx" 和 "reportlab" 来实现将 word 转成 pdf 的功能。
代码如下:
```
from docx import Document
from docx.shared import Inches
from docx2pdf import convert
# 加载 word 文档
document = Document("example.docx")
# 转换为 pdf
convert(document, "example.pdf")
print("转换成功!")
```
需要注意的是,在使用此代码之前,需要先安装 "python-docx" 和 "reportlab" 两个库。
相关问题
python 写一个pdf 转word 代码
你可以使用 `pdfminer` 库来解析 pdf 文件,并使用 `python-docx` 库来创建 word 文档。以下是一个简单的示例代码:
```
import io
import os
import docx
from pdfminer.converter import TextConverter
from pdfminer.layout import LAParams
from pdfminer.pdfinterp import PDFResourceManager, PDFPageInterpreter
from pdfminer.pdfpage import PDFPage
def pdf_to_docx(pdf_path, docx_path):
# 读取 pdf 文件
with open(pdf_path, 'rb') as pdf_file:
resource_manager = PDFResourceManager()
output_string = io.StringIO()
codec = 'utf-8'
layout_params = LAParams()
converter = TextConverter(resource_manager, output_string, codec=codec, laparams=layout_params)
interpreter = PDFPageInterpreter(resource_manager, converter)
for page in PDFPage.get_pages(pdf_file, check_extractable=True):
interpreter.process_page(page)
text = output_string.getvalue()
converter.close()
output_string.close()
# 创建 word 文档
doc = docx.Document()
for line in text.split('\n'):
doc.add_paragraph(line)
# 保存 word 文档
doc.save(docx_path)
# 使用示例
pdf_to_docx('example.pdf', 'example.docx')
```
这个函数将读取名为 `example.pdf` 的 pdf 文件,并将其转换为名为 `example.docx` 的 word 文档。你可以根据需要修改文件名和路径。
用python写一段pdf转word代码
我可以回答这个问题。你可以使用Python中的第三方库PyPDF2来读取PDF文件,然后使用Python-docx库将PDF内容转换为Word文档。以下是示例代码:
```
import PyPDF2
from docx import Document
pdf_file = open('example.pdf', 'rb')
pdf_reader = PyPDF2.PdfFileReader(pdf_file)
document = Document()
for page_num in range(pdf_reader.numPages):
page = pdf_reader.getPage(page_num)
text = page.extractText()
document.add_paragraph(text)
document.save('example.docx')
```
请注意,这只是一个示例代码,实际上,PDF转Word可能需要更复杂的代码,具体取决于PDF的结构和内容。
相关推荐
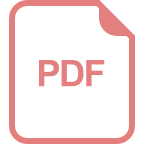
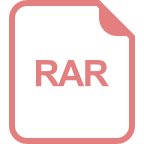
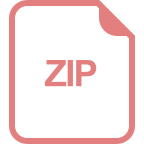












