vue2计算盒子的高度
时间: 2023-09-30 22:12:38 浏览: 126
Vue2中计算盒子高度的方法主要有两种:
1. 使用ref获取dom元素,通过clientHeight或offsetHeight属性获取盒子高度,如下所示:
```
<template>
<div ref="box"></div>
</template>
<script>
export default {
mounted() {
const boxHeight = this.$refs.box.clientHeight;
}
}
</script>
```
2. 使用computed属性计算盒子高度。先定义一个data属性保存内容高度,然后使用计算属性根据内容高度动态计算盒子高度,如下所示:
```
<template>
<div :style="{ height: boxHeight }"></div>
</template>
<script>
export default {
data() {
return {
contentHeight: 200
}
},
computed: {
boxHeight() {
return this.contentHeight + 'px';
}
}
}
</script>
```
希望能对你有所帮助!
相关问题
vue 拿到盒子的高度
在Vue.js中,如果你想要获取元素的高度,通常会通过JavaScript操作DOM或者使用Vue提供的响应式特性。这里有几个常用的方法:
1. **直接访问**:
可以使用`ref`属性创建一个引用,然后在模板中绑定这个引用并读取其高度。例如:
```html
<div ref="myBox"></div>
{{ $refs.myBox.offsetHeight }}
```
2. **计算属性**:
如果需要在数据更新时获取高度,可以定义一个计算属性,它依赖于某个值,比如元素的style对象。
```javascript
data() {
return {
boxStyle: null,
}
},
computed: {
boxHeight() {
if (this.boxStyle) {
return this.boxStyle.height;
} else {
// 使用懒加载或者其他方法获取高度
}
}
},
mounted() {
this.boxStyle = window.getComputedStyle(this.$refs.myBox);
}
```
3. **事件监听**:
也可以通过`@update`或自定义事件来获取动态变化的高度。
注意:以上示例假设你有`mounted`生命周期钩子函数用于初始化获取高度。如果元素是在组件渲染后添加到DOM的,记得处理这种情况。
vue3项目 怎么获取页面 div的高度 4个盒子的高度总和,给外边的父盒子
你可以使用 Vue 3 中的 ref 和 reactive 来获取页面 div 的高度。首先,在 Vue 组件中,通过 ref 创建一个对应的引用变量来获取 div 元素的引用。然后,使用 reactive 创建一个响应式对象来保存盒子的高度总和。接下来,可以通过监听 div 元素的变化来更新盒子的高度总和,并将其赋值给父盒子。
以下是一个示例代码:
```vue
<template>
<div>
<div ref="box1">...</div>
<div ref="box2">...</div>
<div ref="box3">...</div>
<div ref="box4">...</div>
<div ref="parentBox" :style="{ height: boxHeight + 'px' }">...</div>
</div>
</template>
<script>
import { ref, reactive, onMounted } from 'vue';
export default {
setup() {
const box1 = ref(null);
const box2 = ref(null);
const box3 = ref(null);
const box4 = ref(null);
const parentBox = ref(null);
const boxHeight = reactive({
totalHeight: 0,
});
const updateBoxHeight = () => {
const height1 = box1.value.offsetHeight;
const height2 = box2.value.offsetHeight;
const height3 = box3.value.offsetHeight;
const height4 = box4.value.offsetHeight;
boxHeight.totalHeight = height1 + height2 + height3 + height4;
};
onMounted(() => {
updateBoxHeight();
window.addEventListener('resize', updateBoxHeight);
});
return {
box1,
box2,
box3,
box4,
parentBox,
boxHeight,
};
},
};
</script>
```
在上面的示例代码中,通过 ref 创建了 `box1`、`box2`、`box3`、`box4` 和 `parentBox` 引用变量,分别对应页面中的四个盒子和父盒子。使用 reactive 创建了一个响应式对象 `boxHeight` 来保存盒子的高度总和。在 `updateBoxHeight` 函数中,通过获取每个盒子的 `offsetHeight` 来计算盒子的高度总和,并将其赋值给 `boxHeight.totalHeight`。在组件的 `setup` 钩子函数中,通过监听窗口的 resize 事件来更新盒子的高度总和。
最后,在父盒子上使用 `:style="{ height: boxHeight + 'px' }"` 来绑定父盒子的高度,实现将盒子的高度总和赋值给父盒子的效果。
阅读全文
相关推荐
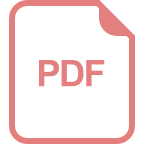
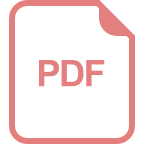
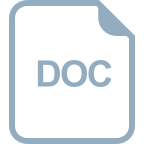
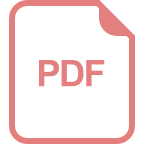
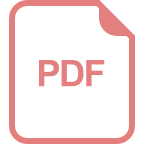
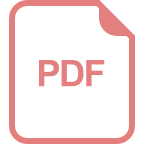
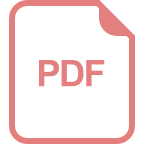
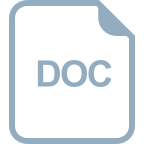








