用c++写以班级为单位进行选题,题目信息以文件形式存放, 包括:编号、题目、任务要求、完成时间 功能要求: 1录入学生信息包括:学号、姓名、班级; 2输入学号,随机抽取题目,限定每个题目只能被一个学生选中 3选题结果以文件形式存放,可以随时进行查询操作。
时间: 2024-02-06 17:02:54 浏览: 33
好的,您的问题是如何用C++编写一个以班级为单位进行选题的程序,包括录入学生信息、随机抽取题目、选题结果存储和查询。
首先,我们需要设计数据结构,包括学生信息和题目信息。对于学生信息,可以定义一个结构体,包括学号、姓名、班级等字段。对于题目信息,也可以定义一个结构体,包括编号、题目、任务要求、完成时间等字段。
然后,我们需要实现程序的功能。首先,我们可以编写一个函数用于录入学生信息,将学生信息存储到文件中。然后,我们可以编写一个函数用于读取题目信息文件,将题目信息存储到一个题库中。接下来,我们可以编写一个函数用于随机抽取题目,限定每个题目只能被一个学生选中。最后,我们可以编写一个函数将选题结果存储到文件中,并且可以随时进行查询操作。
下面是一个简单的示例程序,供您参考:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <cstdlib>
#include <ctime>
using namespace std;
struct Student {
string id;
string name;
string clazz;
};
struct Topic {
string id;
string title;
string requirement;
string deadline;
string chosen_by;
};
vector<Student> students;
vector<Topic> topics;
void read_students(const string& filename) {
ifstream ifs(filename);
if (!ifs) {
cerr << "Failed to open file: " << filename << endl;
exit(1);
}
string line;
while (getline(ifs, line)) {
Student s;
ifs >> s.id >> s.name >> s.clazz;
students.push_back(s);
}
ifs.close();
}
void read_topics(const string& filename) {
ifstream ifs(filename);
if (!ifs) {
cerr << "Failed to open file: " << filename << endl;
exit(1);
}
string line;
while (getline(ifs, line)) {
Topic t;
ifs >> t.id >> t.title >> t.requirement >> t.deadline;
topics.push_back(t);
}
ifs.close();
}
void choose_topic() {
int n = students.size();
int m = topics.size();
srand(time(nullptr));
for (int i = 0; i < m; i++) {
int idx = rand() % n;
while (topics[i].chosen_by != "") {
i = (i + 1) % m;
}
topics[i].chosen_by = students[idx].id;
}
}
void save_result(const string& filename) {
ofstream ofs(filename);
if (!ofs) {
cerr << "Failed to open file: " << filename << endl;
exit(1);
}
for (auto& t : topics) {
ofs << t.id << '\t' << t.chosen_by << endl;
}
ofs.close();
}
void print_topics() {
for (auto& t : topics) {
cout << t.id << '\t' << t.title << '\t' << t.chosen_by << endl;
}
}
int main() {
read_students("students.txt");
read_topics("topics.txt");
choose_topic();
save_result("result.txt");
print_topics();
return 0;
}
```
在这个示例程序中,我们定义了两个结构体 `Student` 和 `Topic`,分别表示学生信息和题目信息。我们使用 STL 中的 `vector` 来存储学生和题目信息,方便进行遍历和查询。
首先,我们编写了一个函数 `read_students`,用于读取学生信息文件,将学生信息存储到 `students` 中。类似地,我们编写了一个函数 `read_topics`,用于读取题目信息文件,将题目信息存储到 `topics` 中。
然后,我们编写了一个函数 `choose_topic`,用于随机抽取题目,并将选题结果存储在 `topics` 中。在这个函数中,我们使用了 `rand` 函数来生成随机数,限定每个题目只能被一个学生选中。
最后,我们编写了一个函数 `save_result`,用于将选题结果存储到文件中。在这个函数中,我们使用了 `ofstream` 类来打开文件,并将选题结果写入文件中。
在 `main` 函数中,我们依次调用了上述函数,完成了程序的各项功能。最后,我们编写了一个函数 `print_topics`,用于打印选题结果,方便进行查询操作。
相关推荐
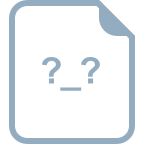












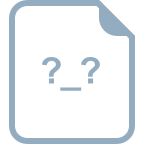
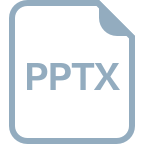
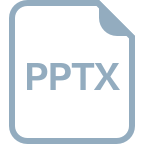